贪吃蛇代码用java怎么写
时间: 2024-01-02 16:01:37 浏览: 62
以下是一个简单的贪吃蛇代码示例,用Java编写:
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Snake extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private final int WIDTH = 500;
private final int HEIGHT = 500;
private Timer timer;
private int delay = 100;
private Image ball;
private Image head;
private Image tail;
private int snakeLength = 3;
private int[] x = new int[500];
private int[] y = new int[500];
private int foodX;
private int foodY;
private boolean left = false;
private boolean right = true;
private boolean up = false;
private boolean down = false;
private boolean gameover = false;
public Snake() {
setTitle("贪吃蛇");
setSize(WIDTH, HEIGHT);
setResizable(false);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if ((key == KeyEvent.VK_LEFT) && (!right)) {
left = true;
up = false;
down = false;
}
if ((key == KeyEvent.VK_RIGHT) && (!left)) {
right = true;
up = false;
down = false;
}
if ((key == KeyEvent.VK_UP) && (!down)) {
up = true;
right = false;
left = false;
}
if ((key == KeyEvent.VK_DOWN) && (!up)) {
down = true;
right = false;
left = false;
}
}
});
ImageIcon icon1 = new ImageIcon("ball.png");
ball = icon1.getImage();
ImageIcon icon2 = new ImageIcon("head.png");
head = icon2.getImage();
ImageIcon icon3 = new ImageIcon("tail.png");
tail = icon3.getImage();
initGame();
}
public void initGame() {
snakeLength = 3;
x[0] = 100;
y[0] = 100;
x[1] = 80;
y[1] = 100;
x[2] = 60;
y[2] = 100;
generateFood();
timer = new Timer(delay, this);
timer.start();
}
public void generateFood() {
int randX = (int) (Math.random() * (WIDTH - 20) + 10);
int randY = (int) (Math.random() * (HEIGHT - 20) + 10);
foodX = randX - (randX % 10);
foodY = randY - (randY % 10);
}
public void checkFood() {
if ((x[0] == foodX) && (y[0] == foodY)) {
snakeLength++;
generateFood();
}
}
public void checkCollision() {
for (int i = snakeLength; i > 0; i--) {
if ((i > 4) && (x[0] == x[i]) && (y[0] == y[i])) {
gameover = true;
}
}
if (y[0] >= HEIGHT) {
gameover = true;
}
if (y[0] < 0) {
gameover = true;
}
if (x[0] >= WIDTH) {
gameover = true;
}
if (x[0] < 0) {
gameover = true;
}
if(gameover) {
timer.stop();
}
}
public void move() {
for (int i = snakeLength; i > 0; i--) {
x[i] = x[(i - 1)];
y[i] = y[(i - 1)];
}
if (left) {
x[0] -= 10;
}
if (right) {
x[0] += 10;
}
if (up) {
y[0] -= 10;
}
if (down) {
y[0] += 10;
}
}
public void paint(Graphics g) {
super.paint(g);
Graphics2D g2 = (Graphics2D) g;
if (!gameover) {
g2.drawImage(ball, foodX, foodY, this);
for (int i = 0; i < snakeLength; i++) {
if (i == 0) {
g2.drawImage(head, x[i], y[i], this);
} else if (i == snakeLength - 1) {
g2.drawImage(tail, x[i], y[i], this);
} else {
g2.drawImage(ball, x[i], y[i], this);
}
}
} else {
g2.drawString("游戏结束", WIDTH / 2 - 50, HEIGHT / 2);
}
}
public void actionPerformed(ActionEvent e) {
if (!gameover) {
checkFood();
checkCollision();
move();
}
repaint();
}
public static void main(String[] args) {
new Snake().setVisible(true);
}
}
阅读全文
相关推荐
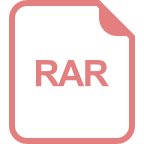
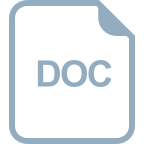
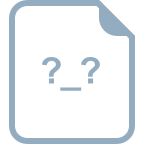
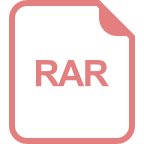
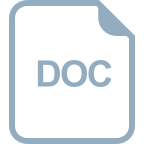
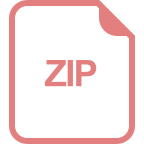
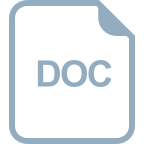
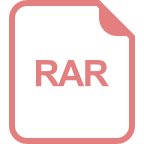






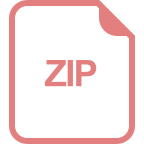