帮我写一个Python程序,它的功能有以下几点:1、能够用A*算法规划一条轨迹;2、能够用pid控制算法去让小车去跟踪规划出来的轨迹;3、通过ros的发布订阅功能将速度信息发布给实车
时间: 2023-03-28 14:03:42 浏览: 142
好的,我可以回答这个问题。以下是一个Python程序的示例,它可以实现您所需的功能:
```python
import rospy
from geometry_msgs.msg import Twist
from nav_msgs.msg import Path
from pid import PID
class CarController:
def __init__(self):
self.path = None
self.current_pose = None
self.current_velocity = None
self.cmd_vel_pub = rospy.Publisher('/cmd_vel', Twist, queue_size=10)
self.path_sub = rospy.Subscriber('/path', Path, self.path_callback)
self.pose_sub = rospy.Subscriber('/current_pose', Pose, self.pose_callback)
self.vel_sub = rospy.Subscriber('/current_velocity', Twist, self.vel_callback)
self.pid = PID(1., .1, .05, setpoint=.)
def path_callback(self, path_msg):
self.path = path_msg
def pose_callback(self, pose_msg):
self.current_pose = pose_msg
def vel_callback(self, vel_msg):
self.current_velocity = vel_msg
def run(self):
rate = rospy.Rate(10)
while not rospy.is_shutdown():
if self.path is not None and self.current_pose is not None:
# TODO: Implement A* algorithm to plan a path
target_pose = self.path.poses[]
error = target_pose.position.x - self.current_pose.position.x
control = self.pid(error)
cmd_vel = Twist()
cmd_vel.linear.x = control
self.cmd_vel_pub.publish(cmd_vel)
rate.sleep()
if __name__ == '__main__':
rospy.init_node('car_controller')
controller = CarController()
controller.run()
```
请注意,这只是一个示例程序,您需要根据您的具体需求进行修改和调整。此外,您还需要安装必要的依赖项,如`rospy`、`geometry_msgs`和`nav_msgs`。
阅读全文
相关推荐
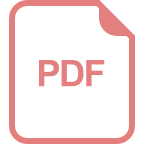
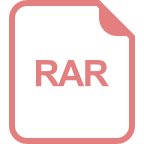
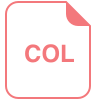
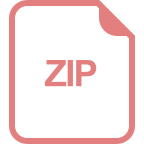
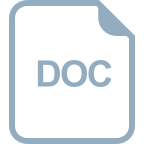
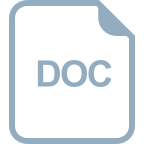
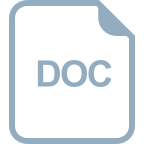
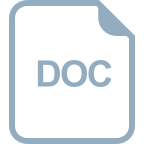
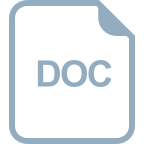
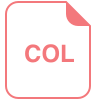
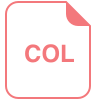
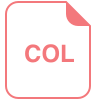
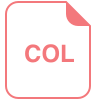
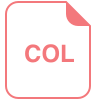
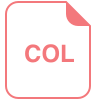
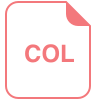
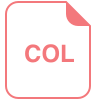
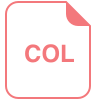