php curl 模拟多线程
时间: 2023-09-06 20:10:01 浏览: 139
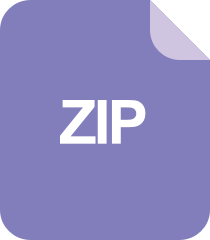
php利用curl实现多线程下载图片类
要使用 PHP 模拟多线程发送 curl 请求,可以使用多进程或多线程的方式来实现。这里介绍两种方式:
1.使用多进程
使用 PHP 的 pcntl_fork() 函数可以创建一个子进程,让子进程去执行 curl 请求,从而达到模拟多线程的效果。代码示例:
```
<?php
$urls = [
'http://www.example.com',
'http://www.example.net',
'http://www.example.org',
];
foreach ($urls as $url) {
$pid = pcntl_fork(); // 创建子进程
if ($pid == -1) {
die('fork failed');
} elseif ($pid) {
// 父进程,继续执行
} else {
// 子进程,执行 curl 请求
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$output = curl_exec($ch);
curl_close($ch);
echo $output; // 处理 curl 响应
exit(); // 子进程退出
}
}
// 等待所有子进程完成
while (pcntl_waitpid(0, $status) != -1) {
$status = pcntl_wexitstatus($status);
echo "Child $status completed\n";
}
```
上述代码将创建多个子进程,每个子进程执行一个 curl 请求,完成后退出。父进程则继续等待所有子进程完成后结束。
2.使用多线程
使用 PHP 的 pthreads 扩展可以实现多线程。代码示例:
```
<?php
class CurlThread extends Thread
{
public $url;
public $result;
public function __construct($url)
{
$this->url = $url;
}
public function run()
{
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $this->url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$this->result = curl_exec($ch);
curl_close($ch);
}
}
$urls = [
'http://www.example.com',
'http://www.example.net',
'http://www.example.org',
];
$threads = [];
foreach ($urls as $url) {
$thread = new CurlThread($url);
$threads[] = $thread;
$thread->start(); // 启动线程
}
foreach ($threads as $thread) {
$thread->join(); // 等待线程完成
echo $thread->result; // 处理线程的 curl 响应
}
```
上述代码将创建多个线程,每个线程执行一个 curl 请求,完成后处理响应。线程的执行顺序不确定,但是由于每个线程都是独立的,所以可以实现真正的多线程效果。
阅读全文
相关推荐
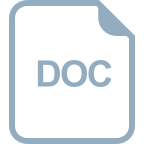
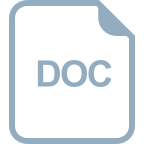
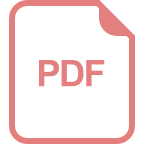
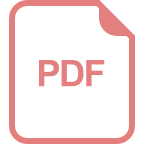
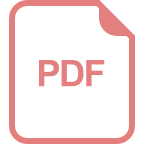
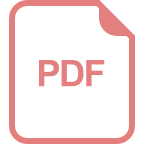
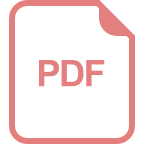
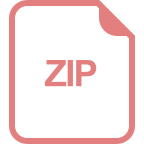
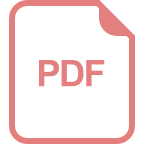
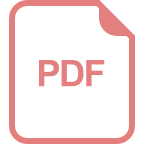
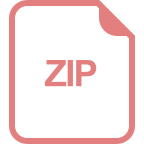
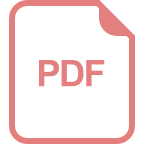
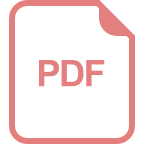
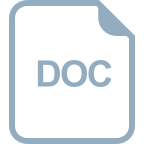
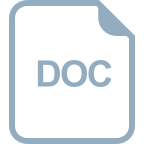
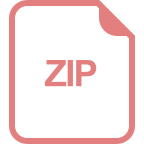
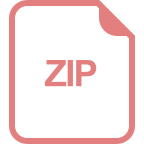