C语言实现fly happy bird
时间: 2024-03-25 08:40:21 浏览: 140
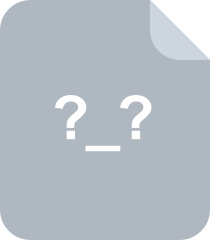
C语言版flappy bird
Fly Happy Bird(飞行的快乐小鸟)是一款非常经典的小游戏,使用 C 语言可以实现类似的游戏效果。下面简单介绍一下实现的过程。
1. 绘制游戏界面
首先需要绘制游戏界面,包括背景、小鸟、障碍物等。可以使用图形库(如 graphics.h)或者游戏引擎(如 SDL、OpenGL 等)来绘制游戏界面。
2. 实现小鸟的飞行
小鸟的飞行可以通过修改小鸟的纵坐标实现。可以使用定时器或者循环来控制小鸟的飞行速度和高度。
3. 实现障碍物的移动和碰撞检测
障碍物可以使用随机数生成,然后通过移动来实现障碍物的移动。同时需要实现碰撞检测,当小鸟和障碍物发生碰撞时,游戏结束。
4. 实现分数统计和游戏结束
当小鸟经过一个障碍物时,可以增加分数统计。同时需要判断游戏是否结束,当小鸟和障碍物发生碰撞或者小鸟飞出屏幕时,游戏结束。
下面是一个简单的示例代码,使用图形库实现 Fly Happy Bird 游戏:
```c++
#include <graphics.h>
#include <conio.h>
#include <time.h>
#define WIDTH 640
#define HEIGHT 480
#define BIRD_SIZE 40
#define PIPE_WIDTH 50
#define PIPE_GAP 150
#define PIPE_SPEED 5
#define GRAVITY 1
// 小鸟结构体
struct Bird
{
int x, y;
int vy;
};
// 障碍物结构体
struct Pipe
{
int x;
int y1, y2;
};
// 绘制小鸟
void drawBird(Bird bird)
{
setfillcolor(YELLOW);
fillcircle(bird.x, bird.y, BIRD_SIZE / 2);
}
// 绘制障碍物
void drawPipe(Pipe pipe)
{
setfillcolor(GREEN);
bar(pipe.x, 0, pipe.x + PIPE_WIDTH, pipe.y1);
bar(pipe.x, pipe.y2, pipe.x + PIPE_WIDTH, HEIGHT);
}
// 随机生成障碍物
Pipe generatePipe()
{
Pipe pipe;
pipe.x = WIDTH;
int gap = rand() % (HEIGHT - PIPE_GAP);
pipe.y1 = gap;
pipe.y2 = gap + PIPE_GAP;
return pipe;
}
// 检测碰撞
bool checkCollision(Bird bird, Pipe pipe)
{
if (bird.x > pipe.x && bird.x < pipe.x + PIPE_WIDTH)
{
if (bird.y < pipe.y1 || bird.y > pipe.y2)
{
return true;
}
}
return false;
}
int main()
{
srand((unsigned)time(NULL));
initgraph(WIDTH, HEIGHT);
Bird bird;
bird.x = WIDTH / 2;
bird.y = HEIGHT / 2;
bird.vy = 0;
Pipe pipe = generatePipe();
int score = 0;
while (true)
{
// 绘制游戏界面
cleardevice();
drawBird(bird);
drawPipe(pipe);
outtextxy(10, 10, std::to_string(score).c_str());
Sleep(30);
// 处理用户输入
if (_kbhit())
{
char ch = _getch();
if (ch == ' ')
{
bird.vy = -10;
}
}
// 更新小鸟位置
bird.vy += GRAVITY;
bird.y += bird.vy;
// 更新障碍物位置
pipe.x -= PIPE_SPEED;
if (pipe.x <= -PIPE_WIDTH)
{
pipe = generatePipe();
score++;
}
// 检测碰撞
if (bird.y > HEIGHT || checkCollision(bird, pipe))
{
outtextxy(WIDTH / 2 - 50, HEIGHT / 2 - 10, "Game Over");
break;
}
}
getch();
closegraph();
return 0;
}
```
这个示例代码使用了图形库来绘制游戏界面,并且通过键盘输入来控制小鸟的飞行。障碍物通过随机数生成,然后通过移动来实现移动效果。碰撞检测使用了简单的矩形碰撞检测。同时还实现了分数统计和游戏结束的逻辑。
阅读全文
相关推荐

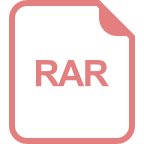
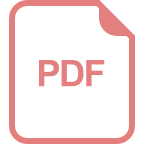
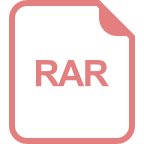
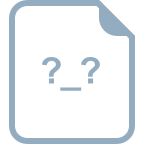
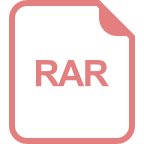
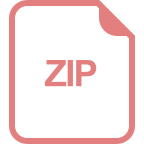
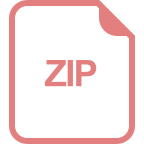
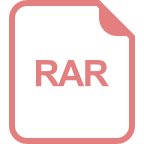
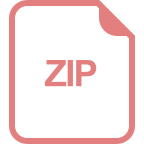