java 网络文件链接下载到本地,然后转换成文件,获取文件二进制数据再进行base64编码,再删除本地文件
时间: 2024-09-11 15:12:58 浏览: 17
在Java中,你可以使用`java.net.URL`、`java.io.InputStream`以及相关的I/O流来下载网络文件,然后将内容读取为字节,接着进行Base64编码。最后,如果你需要删除这个临时文件,可以使用`File`类的delete()方法。这里是一个简单的示例:
```java
import java.io.*;
import java.net.URL;
public class FileDownloadAndEncoding {
public static void main(String[] args) throws IOException {
// 创建URL对象
URL url = new URL("http://example.com/file.zip"); // 替换为你想要下载的文件地址
// 创建文件存储路径
String localFilePath = "tempFile.zip";
// 下载并保存到本地
try (InputStream in = url.openStream();
FileOutputStream fileOutputStream = new FileOutputStream(localFilePath)) {
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
fileOutputStream.write(buffer, 0, bytesRead);
}
System.out.println("文件已下载到本地");
}
// 读取文件内容为字节数组
FileInputStream fis = new FileInputStream(localFilePath);
byte[] bytes = new byte[(int) fis.getChannel().size()];
fis.read(bytes);
// 关闭输入流
fis.close();
// 对字节数组进行Base64编码
String base64Encoded = Base64.getEncoder().encodeToString(bytes);
System.out.println("Base64编码后的字符串: " + base64Encoded);
// 删除本地文件
boolean deleted = new File(localFilePath).delete();
if (deleted) {
System.out.println("本地文件已删除");
} else {
System.out.println("删除文件失败");
}
}
}
```
在这个例子中,注意处理异常,因为网络连接和文件操作都有可能出现错误。同时,记得替换URL地址和文件名。
相关推荐
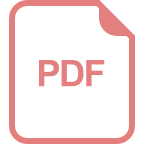
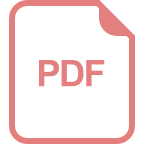
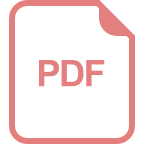














