用Java语句创建MySQL表,并实现增、查、并利用触发器
时间: 2023-12-26 18:05:29 浏览: 94
好的,以下是用Java语句创建MySQL表的示例代码:
```java
import java.sql.*;
public class CreateTable {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/TESTDB";
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try{
Class.forName("com.mysql.jdbc.Driver");
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL,USER,PASS);
System.out.println("Creating table...");
stmt = conn.createStatement();
String sql = "CREATE TABLE STUDENTS " +
"(id INTEGER not NULL, " +
" name VARCHAR(255), " +
" age INTEGER, " +
" PRIMARY KEY ( id ))";
stmt.executeUpdate(sql);
System.out.println("Table created successfully...");
stmt.close();
conn.close();
}catch(SQLException se){
se.printStackTrace();
}catch(Exception e){
e.printStackTrace();
}finally{
try{
if(stmt!=null)
stmt.close();
}catch(SQLException se2){
}
try{
if(conn!=null)
conn.close();
}catch(SQLException se){
se.printStackTrace();
}
}
System.out.println("End of program...");
}
}
```
这个示例代码会连接到本地MySQL数据库的TESTDB数据库,并且创建一个名为STUDENTS的表,包含id、name和age三个字段,其中id为主键。
接下来,我们可以使用下面的代码实现增、查操作:
```java
import java.sql.*;
public class InsertSelect {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/TESTDB";
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try{
Class.forName("com.mysql.jdbc.Driver");
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL,USER,PASS);
System.out.println("Inserting records into the table...");
stmt = conn.createStatement();
String sql = "INSERT INTO STUDENTS " +
"VALUES (100, 'Zara', 18)";
stmt.executeUpdate(sql);
sql = "INSERT INTO STUDENTS " +
"VALUES (101, 'Mahnaz', 22)";
stmt.executeUpdate(sql);
sql = "INSERT INTO STUDENTS " +
"VALUES (102, 'Zaid', 19)";
stmt.executeUpdate(sql);
sql = "INSERT INTO STUDENTS " +
"VALUES(103, 'Sumit', 20)";
stmt.executeUpdate(sql);
System.out.println("Records inserted successfully...");
System.out.println("Fetching records from the table...");
sql = "SELECT id, name, age FROM STUDENTS";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.print("ID: " + id);
System.out.print(", Name: " + name);
System.out.print(", Age: " + age);
System.out.println();
}
rs.close();
stmt.close();
conn.close();
}catch(SQLException se){
se.printStackTrace();
}catch(Exception e){
e.printStackTrace();
}finally{
try{
if(stmt!=null)
stmt.close();
}catch(SQLException se2){
}
try{
if(conn!=null)
conn.close();
}catch(SQLException se){
se.printStackTrace();
}
}
System.out.println("End of program...");
}
}
```
这个示例代码会向STUDENTS表中插入四条记录,并且查询所有记录并输出。
最后,我们可以使用下面的代码实现一个简单的触发器,当向STUDENTS表中插入新记录时,自动向另一个表中插入一条记录:
```java
import java.sql.*;
public class TriggerExample {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/TESTDB";
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try{
Class.forName("com.mysql.jdbc.Driver");
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL,USER,PASS);
System.out.println("Creating trigger...");
stmt = conn.createStatement();
String sql = "CREATE TRIGGER insert_record " +
"AFTER INSERT ON STUDENTS " +
"FOR EACH ROW " +
"INSERT INTO STUDENTS_LOG " +
"VALUES (new.id, new.name, new.age)";
stmt.executeUpdate(sql);
System.out.println("Trigger created successfully...");
System.out.println("Inserting records into the table...");
stmt = conn.createStatement();
sql = "INSERT INTO STUDENTS " +
"VALUES (104, 'John', 21)";
stmt.executeUpdate(sql);
System.out.println("Record inserted successfully...");
System.out.println("Fetching records from the table...");
sql = "SELECT id, name, age FROM STUDENTS_LOG";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.print("ID: " + id);
System.out.print(", Name: " + name);
System.out.print(", Age: " + age);
System.out.println();
}
rs.close();
stmt.close();
conn.close();
}catch(SQLException se){
se.printStackTrace();
}catch(Exception e){
e.printStackTrace();
}finally{
try{
if(stmt!=null)
stmt.close();
}catch(SQLException se2){
}
try{
if(conn!=null)
conn.close();
}catch(SQLException se){
se.printStackTrace();
}
}
System.out.println("End of program...");
}
}
```
这个示例代码会创建一个名为insert_record的触发器,当向STUDENTS表中插入新记录时,自动向STUDENTS_LOG表中插入一条记录,然后插入一条新记录并查询STUDENTS_LOG表中的所有记录并输出。
需要注意的是,这些示例代码中的数据库连接参数需要根据实际情况进行修改。
阅读全文
相关推荐
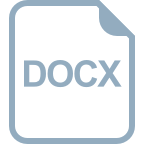
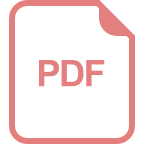
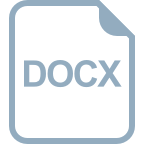















