用c++语言写一个简单的rpg游戏
时间: 2023-11-12 22:05:35 浏览: 48
好的,这里给您提供一个简单的RPG游戏示例代码,您可以参考一下:
```c++
#include <iostream>
#include <string>
#include <ctime>
using namespace std;
// 角色类
class Role
{
public:
Role(string n, int hp, int atk, int def)
: name(n), maxHp(hp), hp(hp), atk(atk), def(def)
{
}
void show()
{
cout << "------------------" << endl;
cout << "角色名:" << name << endl;
cout << "生命值:" << hp << "/" << maxHp << endl;
cout << "攻击力:" << atk << endl;
cout << "防御力:" << def << endl;
cout << "------------------" << endl;
}
protected:
string name; // 名称
int maxHp; // 最大生命值
int hp; // 当前生命值
int atk; // 攻击力
int def; // 防御力
};
// 怪物类
class Monster
{
public:
Monster(string n, int hp, int atk, int def)
: name(n), maxHp(hp), hp(hp), atk(atk), def(def)
{
}
void show()
{
cout << "------------------" << endl;
cout << "怪物名:" << name << endl;
cout << "生命值:" << hp << "/" << maxHp << endl;
cout << "攻击力:" << atk << endl;
cout << "防御力:" << def << endl;
cout << "------------------" << endl;
}
protected:
string name; // 名称
int maxHp; // 最大生命值
int hp; // 当前生命值
int atk; // 攻击力
int def; // 防御力
};
// 战斗类
class Battle
{
public:
static void start(Role &role, Monster &monster)
{
cout << "战斗开始!" << endl;
while (role.hp > 0 && monster.hp > 0)
{
int roleDamage = role.atk - monster.def;
if (roleDamage <= 0)
roleDamage = 1;
int monsterDamage = monster.atk - role.def;
if (monsterDamage <= 0)
monsterDamage = 1;
cout << "你对" << monster.name << "造成了" << roleDamage << "点伤害!" << endl;
monster.hp -= roleDamage;
if (monster.hp <= 0)
{
cout << "你打败了" << monster.name << ",获得胜利!" << endl;
break;
}
cout << monster.name << "对你造成了" << monsterDamage << "点伤害!" << endl;
role.hp -= monsterDamage;
if (role.hp <= 0)
{
cout << "你被" << monster.name << "打败了,游戏结束!" << endl;
break;
}
}
role.show();
monster.show();
}
};
int main()
{
srand(time(nullptr));
Role role("勇者", 100, 10, 5);
role.show();
Monster monster("史莱姆", 50, 5, 2);
monster.show();
Battle::start(role, monster);
return 0;
}
```
这个游戏非常简单,只有一个角色和一个怪物,但是包含了基本的战斗逻辑。在游戏开始时,你会看到角色和怪物的属性信息,然后进入战斗。在战斗中,角色和怪物轮流攻击,每次攻击后都会显示对方的剩余生命值,直到其中一方生命值降为零为止。最后会显示战斗结束后的角色和怪物属性信息。
相关推荐
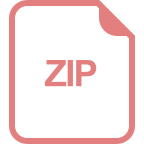
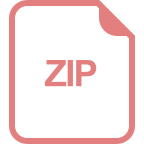
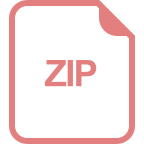












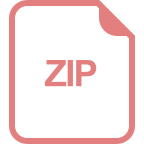
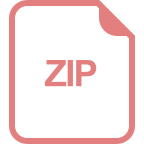
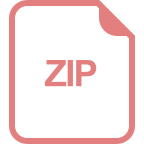