用vue.js语法写一个购物车界面包含功能
时间: 2023-10-09 19:08:15 浏览: 92
好的,下面是一个简单的购物车界面示例,包括添加商品、删除商品、计算总价等功能,使用Vue.js语法:
HTML:
```html
<div id="app">
<h2>购物车</h2>
<table>
<thead>
<tr>
<th>商品名称</th>
<th>单价</th>
<th>数量</th>
<th>小计</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in cartList" :key="index">
<td>{{ item.name }}</td>
<td>{{ item.price }}</td>
<td>
<button @click="decrease(index)">-</button>
{{ item.count }}
<button @click="increase(index)">+</button>
</td>
<td>{{ item.price * item.count }}</td>
<td>
<button @click="remove(index)">删除</button>
</td>
</tr>
</tbody>
<tfoot>
<tr>
<td colspan="3">总价:</td>
<td>{{ totalPrice }}</td>
<td></td>
</tr>
</tfoot>
</table>
<div>
<label>商品名称:</label>
<input v-model="newItem.name">
<label>单价:</label>
<input v-model="newItem.price">
<button @click="addItem">添加商品</button>
</div>
</div>
```
JavaScript:
```js
var app = new Vue({
el: '#app',
data: {
cartList: [
{ name: '商品1', price: 10, count: 2 },
{ name: '商品2', price: 20, count: 1 },
{ name: '商品3', price: 30, count: 3 }
],
newItem: { name: '', price: '' }
},
methods: {
increase(index) {
this.cartList[index].count++;
},
decrease(index) {
if (this.cartList[index].count > 1) {
this.cartList[index].count--;
} else {
this.remove(index);
}
},
remove(index) {
this.cartList.splice(index, 1);
},
addItem() {
if (this.newItem.name && this.newItem.price) {
this.cartList.push({
name: this.newItem.name,
price: this.newItem.price,
count: 1
});
this.newItem.name = '';
this.newItem.price = '';
}
}
},
computed: {
totalPrice() {
var total = 0;
this.cartList.forEach(function(item) {
total += item.price * item.count;
});
return total;
}
}
});
```
这个示例中,我们使用了Vue.js的模板语法,通过v-for指令遍历购物车列表中的每一项,并通过v-model指令实现双向数据绑定。我们还定义了一些方法来处理购物车列表中的商品,例如增加数量、减少数量、删除商品和计算总价等。最后,我们通过computed属性计算总价,并在模板中显示出来。
阅读全文
相关推荐
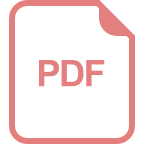
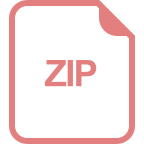
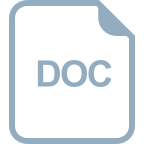
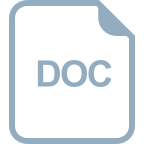
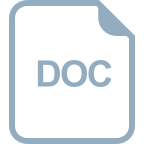

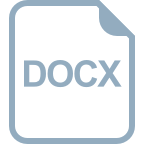
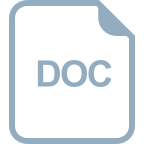
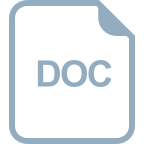
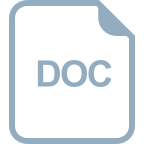
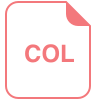
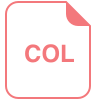
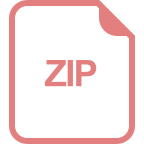
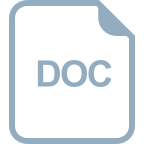
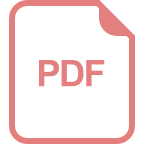
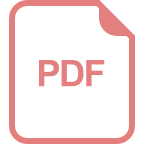
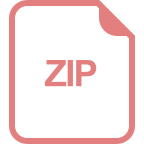
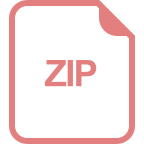