使用for循环依次读取bin文件所有字节并输出为十六进制文本文件的python脚本代码
时间: 2024-12-11 19:44:04 浏览: 15
在Python中,你可以使用`open()`函数打开二进制文件,然后配合`struct`模块解析每个字节,并转换成十六进制表示。下面是一个简单的脚本示例:
```python
# 打开二进制文件,以rb模式读取(b代表二进制)
with open('input.bin', 'rb') as bin_file:
# 使用for循环遍历文件的字节
for byte in bin_file.read():
# 将字节转换为十六进制并打印
hex_value = format(byte, '02x')
print(hex_value, end=' ') # 输出每个字节前加上空格分隔
# 如果你想将结果保存到另一个文本文件,可以这样做
hex_output_file = open('output.txt', 'w')
hex_output_file.write('\n'.join(hex_value for byte in bin_file.read()))
hex_output_file.close()
```
这个脚本会逐个读取二进制文件中的字节,将其转换为十六进制形式,然后输出到控制台或新的文本文件中。注意,`end=' '`使得输出的字节之间用空格分隔,如果你想换行输出,只需去掉这一行。
相关问题
使用for循环读取同目录下的bin文件所有字节并输出为十六进制文本文件的python脚本代码
可以使用Python的内置模块`os`和`struct`来实现这个功能。下面是一个简单的示例脚本,它遍历指定目录下的所有`.bin`文件,并将它们的内容转换为十六进制格式写入新的文本文件:
```python
import os
import struct
def hexify_bin_files(directory):
# 遍历指定目录及其子目录下的bin文件
for root, dirs, files in os.walk(directory):
for file in files:
if file.endswith('.bin'):
# 获取文件路径
file_path = os.path.join(root, file)
try:
with open(file_path, 'rb') as bin_file:
# 逐块读取二进制数据
byte_block = bin_file.read(1024) # 可调整读取块大小
while byte_block:
# 转换为十六进制字符串并写入新文件
hex_output = ''.join(['%02x ' % ord(b) for b in byte_block])
with open(f'{file_path}_hex.txt', 'a') as hex_file:
hex_file.write(hex_output + '\n')
byte_block = bin_file.read(1024)
except Exception as e:
print(f"Error processing {file_path}: {e}")
# 设置需要处理的目录
directory_to_process = 'your_directory_path'
hexify_bin_files(directory_to_process)
使用for循环逐字节读取同目录下的bin文件并输出为十六进制文本文件的python脚本代码
你可以使用Python的内置函数`open()`和`os`模块来遍历指定目录下的所有bin文件,并使用`struct`库将每个字节转换成十六进制。下面是一个简单的脚本示例:
```python
import os
import struct
# 指定要遍历的目录
directory = '/path/to/your/directory'
# 遍历目录下的bin文件
for filename in os.listdir(directory):
if filename.endswith('.bin'): # 确保是bin文件
file_path = os.path.join(directory, filename)
with open(file_path, 'rb') as bin_file:
while True:
byte = bin_file.read(1) # 逐字节读取
if not byte: # 文件已读完
break
hex_value = '{:02x}'.format(ord(byte)) # 将字节转为十六进制字符串
print(hex_value, end='') # 输出到控制台
# 如果你想把结果保存到另一个十六进制文本文件
# hex_output_path = f'{file_path[:-4]}.hex'
# with open(hex_output_path, 'w') as hex_file:
# hex_file.write('\n'.join(hex_values))
# 提示:在实际操作前,请确认替换'/path/to/your/directory'为你的目标目录路径
```
这个脚本会按行打印出每个.bin文件的十六进制表示,如果需要将其写入单独的文本文件,可以按照注释部分修改。
阅读全文
相关推荐
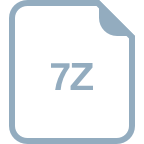
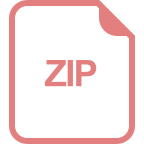
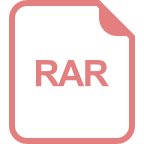













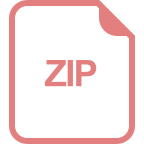