内容2、利用BFS,找到从V1到V6的最短路径(不考虑权重)
时间: 2024-10-22 08:05:05 浏览: 14
在该实验中,您需要使用广度优先搜索(BFS)算法来寻找从顶点 V1 到 V6 的最短路径,这里不考虑边的权重。具体步骤如下:
1. **初始化**:
- 创建一个队列 `Q`,将起始节点 V1 加入队列。
- 创建一个集合 `visited` 来记录已访问的节点,初始时加入 V1。
- 创建一个字典 `parent` 来记录每个节点的父节点,初始时设置 `parent[V1] = None`。
2. **执行 BFS**:
- 当队列 `Q` 不为空时,进行以下操作:
- 从队列 `Q` 中取出第一个节点 `current`。
- 遍历 `current` 的所有邻接节点 `neighbor`:
- 如果 `neighbor` 尚未被访问过(即 `neighbor` 不在 `visited` 集合中),则:
- 将 `neighbor` 标记为已访问,并将其加入 `visited` 集合。
- 设置 `parent[neighbor] = current`。
- 将 `neighbor` 加入队列 `Q`。
3. **构建路径**:
- 从终点 V6 开始,逆向追踪 `parent` 字典,直到到达起点 V1。
- 记录路径上的每个节点。
4. **输出结果**:
- 输出从 V1 到 V6 的最短路径。
### 示例代码
```python
from collections import deque, defaultdict
def bfs_shortest_path(graph, start, end):
# 初始化
queue = deque([start])
visited = set([start])
parent = {start: None}
while queue:
current = queue.popleft()
for neighbor in graph[current]:
if neighbor not in visited:
visited.add(neighbor)
parent[neighbor] = current
queue.append(neighbor)
if neighbor == end:
path = []
node = end
while node is not None:
path.append(node)
node = parent[node]
return list(reversed(path))
return None
# 示例图的邻接表表示
graph = {
'V1': ['V2', 'V3'],
'V2': ['V4'],
'V3': ['V4', 'V5'],
'V4': ['V6'],
'V5': [],
'V6': []
}
# 调用函数
shortest_path = bfs_shortest_path(graph, 'V1', 'V6')
print("从 V1 到 V6 的最短路径:", shortest_path)
```
### 输出
假设图的结构如上所示,运行上述代码后,输出将是:
```
从 V1 到 V6 的最短路径: ['V1', 'V3', 'V4', 'V6']
```
这个路径是从 V1 到 V6 的最短路径,不考虑权重。
阅读全文
相关推荐
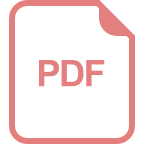
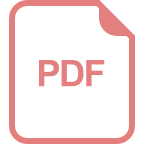
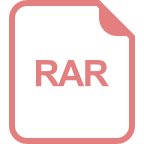
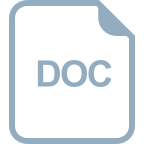
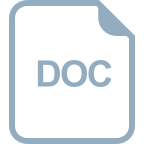
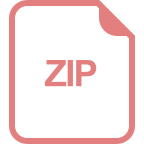
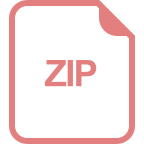
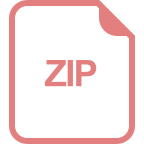
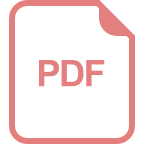
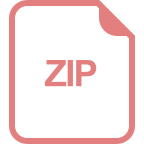
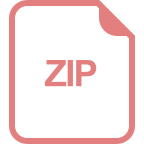