理解布局管理器的使用方法
时间: 2024-01-30 22:40:09 浏览: 90
布局管理器是用来管理组件在容器中的位置和大小的工具。在Java中,常用的布局管理器有FlowLayout、BorderLayout、GridLayout、CardLayout、BoxLayout、GridBagLayout等。
使用布局管理器的一般步骤如下:
1. 创建一个容器(如JPanel)。
2. 创建需要添加到容器中的组件(如JLabel、JButton等)。
3. 将组件添加到容器中。
4. 设置布局管理器(如setLayout(new BorderLayout()))。
5. 设置组件的位置和大小(由布局管理器控制)。
6. 将容器添加到窗口中(如frame.add(panel))。
以FlowLayout为例,下面是一个简单的使用布局管理器的示例代码:
```
import javax.swing.*;
import java.awt.*;
public class LayoutExample extends JFrame {
public LayoutExample() {
setTitle("Layout Example");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 200);
// 创建一个JPanel容器
JPanel panel = new JPanel();
// 创建需要添加到容器中的组件
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
JButton button3 = new JButton("Button 3");
// 将组件添加到容器中
panel.add(button1);
panel.add(button2);
panel.add(button3);
// 设置FlowLayout布局管理器
panel.setLayout(new FlowLayout());
// 将容器添加到窗口中
add(panel);
setVisible(true);
}
public static void main(String[] args) {
new LayoutExample();
}
}
```
在这个例子中,我们创建了一个JPanel容器,并向其中添加了三个JButton组件。然后使用FlowLayout布局管理器将它们排列在一行中。最后将容器添加到窗口中。
需要注意的是,布局管理器可以嵌套使用。比如在一个JPanel容器中使用GridLayout布局管理器,然后在其中添加多个JPanel容器,每个JPanel再使用不同的布局管理器排列组件。这样可以实现更加复杂的布局效果。
阅读全文
相关推荐
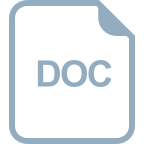
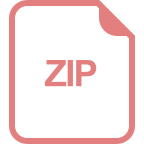
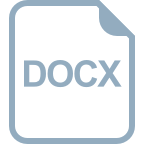
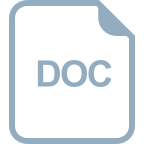
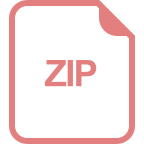
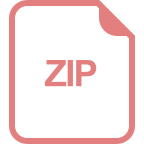
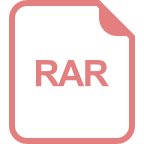
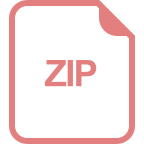
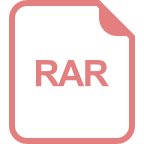
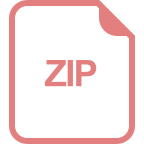
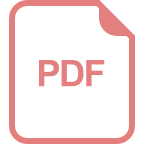
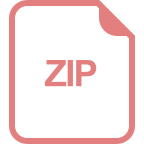
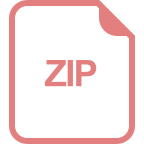
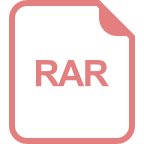