写个俄罗斯方块的python代码
时间: 2023-09-19 13:06:01 浏览: 112
### 回答1:
我提供一个简单的Python代码来实现俄罗斯方块:import pygame
import random #定义屏幕的宽度和高度
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 800#定义屏幕的背景色
WHITE = (255, 255, 255)#定义俄罗斯方块的颜色
BLOCK_COLORS = [(0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255), (0, 255, 255), (255, 255, 255)]#定义俄罗斯方块的格子大小
BLOCK_SIZE = 20 #定义游戏的窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("俄罗斯方块")#定义游戏的主循环
running = True
while running:
#设置背景色
screen.fill(WHITE) #随机生成方块
block_x = random.randint(0, SCREEN_WIDTH - BLOCK_SIZE)
block_y = 0
block_color = random.choice(BLOCK_COLORS) #绘制方块
pygame.draw.rect(screen, block_color, (block_x, block_y, BLOCK_SIZE, BLOCK_SIZE)) #更新屏幕
pygame.display.flip() #监听事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False #退出游戏
pygame.quit()
### 回答2:
俄罗斯方块是一款经典的游戏,下面是一个简单的俄罗斯方块的Python代码实现:
```python
import pygame
import random
# 定义窗口大小及颜色
WINDOW_WIDTH = 800
WINDOW_HEIGHT = 600
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
# 定义方块大小和形状
BLOCK_SIZE = 30
SHAPES = [
[[1, 1, 1, 1]],
[[1, 1], [1, 1]],
[[1, 1, 0], [0, 1, 1]],
[[0, 1, 1], [1, 1, 0]],
[[1, 1, 1], [0, 1, 0]],
[[1, 1, 1], [1, 0, 0]],
[[1, 1, 1], [0, 0, 1]]
]
# 初始化游戏
pygame.init()
# 创建窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("俄罗斯方块")
# 定义方块类
class Block:
def __init__(self):
self.x = WINDOW_WIDTH // 2 - BLOCK_SIZE
self.y = 0
self.shape = random.choice(SHAPES)
self.color = random.randint(1, 7)
# 绘制方块
def draw(self):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j] == 1:
pygame.draw.rect(window, self.get_color(), (self.x + j * BLOCK_SIZE, self.y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 获取方块颜色
def get_color(self):
if self.color == 1:
return (0, 255, 255)
elif self.color == 2:
return (255, 0, 255)
elif self.color == 3:
return (255, 255, 0)
elif self.color == 4:
return (0, 0, 255)
elif self.color == 5:
return (0, 255, 0)
elif self.color == 6:
return (255, 0, 0)
elif self.color == 7:
return (255, 165, 0)
# 方块下落
def fall(self):
self.y += BLOCK_SIZE
# 方块左移
def move_left(self):
self.x -= BLOCK_SIZE
# 方块右移
def move_right(self):
self.x += BLOCK_SIZE
# 游戏主循环
def main():
clock = pygame.time.Clock()
block = Block()
running = True
while running:
clock.tick(10)
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
block.move_left()
elif event.key == pygame.K_RIGHT:
block.move_right()
block.fall()
window.fill(BLACK)
block.draw()
pygame.display.flip()
pygame.quit()
if __name__ == "__main__":
main()
```
这个代码实现了一个简单的俄罗斯方块游戏,包括方块的生成和下落、移动等功能。游戏窗口大小为800x600,方块大小为30x30,包含7种不同的形状和颜色。玩家可以通过键盘左右键来移动方块。游戏循环会不断刷新屏幕并检测是否有退出事件,直到玩家退出游戏为止。
### 回答3:
俄罗斯方块是一款经典的游戏,我们可以用Python来实现它。下面是一个基本的俄罗斯方块游戏的代码示例:
```python
import pygame
import random
# 初始化游戏
pygame.init()
# 创建游戏窗口
screen_width, screen_height = 800, 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("俄罗斯方块")
# 定义方块大小和颜色
block_size = 30
block_colors = [(0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255,255,0), (255,0,255), (0,255,255), (255,165,0)]
# 创建方块类
class Block:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
def draw(self):
pygame.draw.rect(screen, self.color, (self.x, self.y, block_size, block_size))
# 创建游戏区域矩阵
game_matrix = [[(0, 0, 0) for _ in range(screen_width // block_size)] for _ in range(screen_height // block_size)]
# 创建方块对象
def create_block():
x = screen_width // block_size // 2 * block_size
y = 0
color = random.choice(block_colors[1:])
return Block(x, y, color)
# 绘制游戏区域
def draw_game_area():
for row in range(len(game_matrix)):
for col in range(len(game_matrix[row])):
pygame.draw.rect(screen, game_matrix[row][col], (col * block_size, row * block_size, block_size, block_size))
# 判断方块是否触底或碰到其他方块
def check_collision(block):
for row in range(len(game_matrix)):
for col in range(len(game_matrix[row])):
if game_matrix[row][col] != (0, 0, 0):
if (block.x, block.y) == (col * block_size, row * block_size):
return True
return False
# 将方块加入游戏区域矩阵
def add_block_to_game_matrix(block):
for row in range(len(game_matrix)):
for col in range(len(game_matrix[row])):
if (block.x, block.y) == (col * block_size, row * block_size):
game_matrix[row][col] = block.color
# 消除满行
def clear_full_lines():
full_rows = []
for row in range(len(game_matrix)):
if all(game_matrix[row]):
full_rows.append(row)
for row in full_rows:
game_matrix.pop(row)
game_matrix.insert(0, [(0, 0, 0) for _ in range(screen_width // block_size)])
# 游戏主循环
def game_loop():
clock = pygame.time.Clock()
game_over = False
current_block = create_block()
while not game_over:
screen.fill((255, 255, 255))
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
current_block.x -= block_size
if keys[pygame.K_RIGHT]:
current_block.x += block_size
if keys[pygame.K_DOWN]:
current_block.y += block_size
if current_block.y + block_size >= screen_height or check_collision(current_block):
add_block_to_game_matrix(current_block)
current_block = create_block()
current_block.y += block_size
clear_full_lines()
draw_game_area()
current_block.draw()
pygame.display.update()
clock.tick(10)
pygame.quit()
# 启动游戏
game_loop()
```
这个代码示例实现了一个简单的俄罗斯方块游戏,包括方块的移动、碰撞检测、消行等功能。你可以通过上下左右键控制方块的移动,当方块触底或碰到其他方块时会自动加入到游戏区域矩阵中,当一行方块都被填满时会自动消除并得分。运行上述代码即可开始游戏。
阅读全文
相关推荐
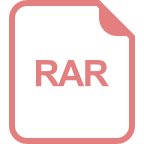
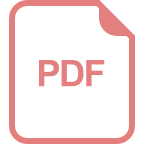

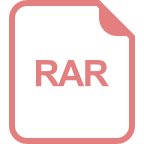
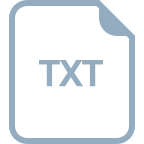
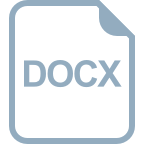
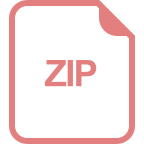
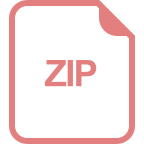
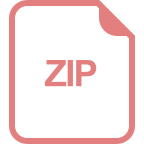
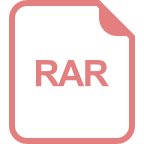
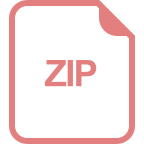
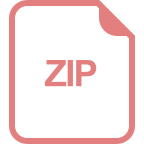
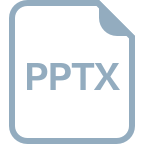