vue3的项目 后台管理系统 角色管理 配置路由权限 操作权限怎么写
时间: 2023-12-27 13:04:50 浏览: 57
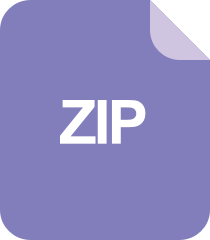
Vue后台管理系统实现权限控制

在 Vue3 的项目中,可以使用 Vue Router 来实现路由权限控制,同时配合使用 Vuex 来实现角色管理和操作权限控制。
1. 定义路由
在定义路由时,可以为每个路由设置一个 meta 属性,用于存储该路由所需要的角色信息。例如:
```javascript
const routes = [
{
path: '/dashboard',
component: Dashboard,
meta: {
roles: ['admin', 'editor']
}
},
{
path: '/user',
component: User,
meta: {
roles: ['admin']
}
}
];
```
2. 定义 Vuex 存储用户信息和角色信息
在 Vuex 中,可以定义一个 user 模块,用于存储用户信息和角色信息。例如:
```javascript
const user = {
state: {
userInfo: null,
roles: []
},
mutations: {
SET_USER_INFO: (state, userInfo) => {
state.userInfo = userInfo;
},
SET_ROLES: (state, roles) => {
state.roles = roles;
}
},
actions: {
getUserInfo({ commit }) {
// 发送请求获取用户信息
// ...
commit('SET_USER_INFO', userInfo);
commit('SET_ROLES', userInfo.roles);
}
}
};
```
3. 判断路由权限
在路由跳转前,可以使用 Vue Router 的 beforeEach 钩子函数判断用户是否有权限访问该路由。在该函数中,可以读取 Vuex 中存储的用户信息和角色信息,与路由的 meta 属性进行比较,判断是否有权限访问该路由。例如:
```javascript
router.beforeEach((to, from, next) => {
const roles = store.state.user.roles;
if (to.meta.roles.includes(roles)) {
next();
} else {
next('/403'); // 跳转到无权限页面
}
});
```
4. 控制操作权限
在界面上,可以根据用户的角色信息来控制操作权限。例如,在按钮上添加 v-if 指令,根据用户角色信息来判断是否显示该按钮。例如:
```html
<template>
<button v-if="hasPermission">
操作按钮
</button>
</template>
<script>
export default {
computed: {
hasPermission() {
const roles = this.$store.state.user.roles;
return roles.includes('admin');
}
}
};
</script>
```
综上所述,通过使用 Vue Router 和 Vuex,可以实现角色管理、路由权限控制和操作权限控制。需要注意的是,在实现时需要注意安全性和灵活性的平衡,同时需要维护好用户角色信息的动态调整。
阅读全文
相关推荐
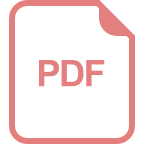
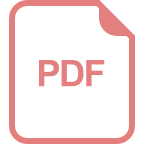















