帮我用keil写一个基于DHT11测量温湿度的stm32f1的c语言程序
时间: 2024-03-16 14:44:51 浏览: 20
好的,以下是一个基于DHT11测量温湿度的STM32F1的C语言程序:
```c
#include "stm32f10x.h"
#define DHT11_PORT GPIOA
#define DHT11_PIN GPIO_Pin_0
void delay_us(uint32_t us) {
uint32_t i = 0;
while (us--) {
i = 10;
while (i--) {};
}
}
void dht11_start(void) {
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
GPIO_ResetBits(DHT11_PORT, DHT11_PIN);
delay_us(18000);
GPIO_SetBits(DHT11_PORT, DHT11_PIN);
delay_us(20);
}
uint8_t dht11_check(void) {
uint8_t retry = 0;
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
while (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) && retry < 100) {
retry++;
delay_us(1);
}
if (retry >= 100) {
return 1;
}
retry = 0;
while (!GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) && retry < 100) {
retry++;
delay_us(1);
}
if (retry >= 100) {
return 1;
}
return 0;
}
uint8_t dht11_read_bit(void) {
uint8_t retry = 0;
while (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) && retry < 100) {
retry++;
delay_us(1);
}
retry = 0;
while (!GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) && retry < 100) {
retry++;
delay_us(1);
}
delay_us(40);
if (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN)) {
return 1;
} else {
return 0;
}
}
uint8_t dht11_read_byte(void) {
uint8_t i, data = 0;
for (i = 0; i < 8; i++) {
data <<= 1;
data |= dht11_read_bit();
}
return data;
}
void dht11_read(uint8_t *temp, uint8_t *hum) {
uint8_t i, buf[5];
dht11_start();
if (dht11_check() == 0) {
for (i = 0; i < 5; i++) {
buf[i] = dht11_read_byte();
}
if ((buf[0] + buf[1] + buf[2] + buf[3]) == buf[4]) {
*hum = buf[0];
*temp = buf[2];
}
}
}
int main(void) {
uint8_t temp, hum;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
while (1) {
dht11_read(&temp, &hum);
printf("Temperature: %dC, Humidity: %d%%\n", temp, hum);
delay_us(500000);
}
}
```
这个程序通过STM32F1的GPIO接口和DHT11传感器进行通信,读取温度和湿度数据,并通过串口输出。请注意,这个程序可能需要根据您的硬件配置进行一些修改。
相关推荐
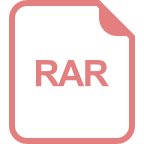
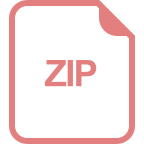
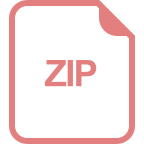
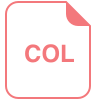
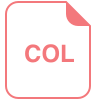
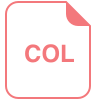
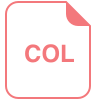
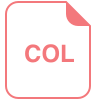









