用python来绘制棋盘格,并用harris算法检测角点,给出完整代码
时间: 2024-02-28 08:27:48 浏览: 17
以下是代码实现:
```python
import cv2
import numpy as np
# 定义棋盘格大小
rows = 8
cols = 8
# 创建棋盘格图像
img = np.zeros((rows*50, cols*50), dtype=np.uint8)
for i in range(0, rows*50, 50):
for j in range(0, cols*50, 50):
if (i//50+j//50) % 2 == 0:
img[i:i+50, j:j+50] = 255
else:
img[i:i+50, j:j+50] = 0
# 使用Harris算法检测角点
gray = np.float32(img)
dst = cv2.cornerHarris(gray, 2, 3, 0.04)
dst = cv2.dilate(dst, None)
# 标记角点
img[dst > 0.01*dst.max()] = 127
# 显示结果
cv2.imshow('Chessboard', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
该代码首先创建一个 $8 \times 8$ 的棋盘格图像,然后使用 Harris 算法检测其中的角点并标记出来,最后显示结果。
相关问题
编写python代码实现用harris算法对棋盘格进行角点检测,并给出完整代码
好的,以下是Python实现的Harris算法检测棋盘格角点的完整代码:
```python
import cv2
img = cv2.imread('chessboard.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 计算Harris角点
dst = cv2.cornerHarris(gray, 2, 3, 0.04)
# 将图像膨胀,以便更清楚地看到角点
dst = cv2.dilate(dst, None)
# 设定阈值,只显示角点
img[dst > 0.01 * dst.max()] = [0, 0, 255]
cv2.imshow('corners', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意,这个代码仅仅是个简单的例子,实际上使用Harris算法进行准确的角点检测还需要进行参数调整和图像处理优化。
希望这个代码对你有所帮助!
用python编写一个绘制棋盘并用Harris()函数进行角点检测的代码
当然,我可以帮你回答这个问题!
首先,我们需要导入必要的库和模块:
```python
import cv2
import numpy as np
```
然后,我们可以定义棋盘的大小和每个方格的大小(这里假设是8x8的棋盘):
```python
board_size = (8,8)
square_size = 50 # 50 pixels
```
接下来,我们可以创建一个棋盘图像的矩阵,并用cv2.rectangle函数绘制每个方格:
```python
board_width = board_size[0] * square_size
board_height = board_size[1] * square_size
board = np.zeros((board_height, board_width), dtype=np.uint8)
for i in range(board_size[0]):
for j in range(board_size[1]):
if (i+j) % 2 == 0:
color = 255
else:
color = 0
x1 = i * square_size
y1 = j * square_size
x2 = (i+1) * square_size
y2 = (j+1) * square_size
cv2.rectangle(board, (x1, y1), (x2, y2), color, -1)
```
现在我们已经创建了棋盘图像。接下来,我们可以使用Harris函数进行角点检测。首先,我们需要将图像转换为灰度,并使用cv2.cornerHarris函数计算角点响应:
```python
gray_board = cv2.cvtColor(board, cv2.COLOR_BGR2GRAY)
dst = cv2.cornerHarris(gray_board, 2, 3, 0.04)
```
然后,我们可以定义一个阈值来找到角点,并使用cv2.findContours函数找到这些角点的位置:
```python
threshold = 0.1 * dst.max()
board[dst > threshold] = [0, 0, 255]
_, contours, hierarchy = cv2.findContours(board, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
```
最后,我们可以在图像上绘制找到的角点:
```python
board_with_corners = board.copy()
for cnt in contours:
x,y,w,h = cv2.boundingRect(cnt)
cv2.rectangle(board_with_corners,(x,y),(x+w,y+h),(0,255,0),2)
```
完整的代码如下:
```python
import cv2
import numpy as np
board_size = (8,8)
square_size = 50 # 50 pixels
board_width = board_size[0] * square_size
board_height = board_size[1] * square_size
board = np.zeros((board_height, board_width), dtype=np.uint8)
for i in range(board_size[0]):
for j in range(board_size[1]):
if (i+j) % 2 == 0:
color = 255
else:
color = 0
x1 = i * square_size
y1 = j * square_size
x2 = (i+1) * square_size
y2 = (j+1) * square_size
cv2.rectangle(board, (x1, y1), (x2, y2), color, -1)
gray_board = cv2.cvtColor(board, cv2.COLOR_BGR2GRAY)
dst = cv2.cornerHarris(gray_board, 2, 3, 0.04)
threshold = 0.1 * dst.max()
board[dst > threshold] = [0, 0, 255]
_, contours, hierarchy = cv2.findContours(board, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
board_with_corners = board.copy()
for cnt in contours:
x,y,w,h = cv2.boundingRect(cnt)
cv2.rectangle(board_with_corners,(x,y),(x+w,y+h),(0,255,0),2)
cv2.imshow("board", board)
cv2.imshow("board with corners", board_with_corners)
cv2.waitKey(0)
```
这个代码应该可以实现你想要的功能。如果有任何问题,请告诉我。
相关推荐
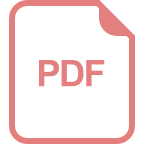
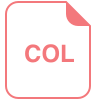
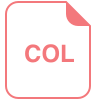
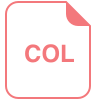









