arduino C语言编写esp8266开发板作为TCP通信的服务器与电脑端python传输数据的代码
时间: 2024-12-14 16:23:54 浏览: 5
在Arduino C语言中,通过ESP8266开发板作为TCP服务器与Python客户端通信,你可以使用`WiFiClient`库处理网络连接,以及`UDPClient`或`TCPServer`用于创建服务器。这里是一个简单的服务器示例:
```c++
#include <ESP8266WiFi.h>
#include <WiFiServer.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
int serverPort = 8080;
WiFiServer server(serverPort);
void setup() {
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
server.begin();
Serial.println("Server started at port " + String(serverPort));
}
void loop() {
WiFiClient client = server.available();
if (!client) {
continue;
}
Serial.println("New connection");
String requestLine = client.readStringUntil('\r');
Serial.println(requestLine);
// 在此处处理接收到的数据请求...
// 这里只是一个基础示例,你需要解析请求并发送回复
String response = "Hello from ESP8266!";
client.print("HTTP/1.1 200 OK\r\n");
client.print("Content-Type: text/plain\r\n");
client.print("Connection: close\r\n\r\n");
client.print(response);
client.stop(); // 关闭连接
}
```
在Python客户端(比如使用`socket`模块),你可以创建一个TCP连接并发送数据。这是一个基本的Python示例:
```python
import socket
def send_data_to_arduino(data):
esp_ip = 'your_ESP_IP' # 替换为你的ESP8266 IP地址
esp_port = 8080
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
sock.connect((esp_ip, esp_port))
sock.sendall(data.encode())
print(f"Sent data: {data}")
response = sock.recv(1024).decode()
print(f"Received from ESP: {response}")
except Exception as e:
print(f"Error: {e}")
finally:
sock.close()
# 使用示例
send_data_to_arduino("Hello from Python!")
```
阅读全文
相关推荐
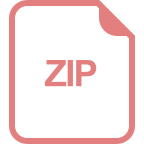
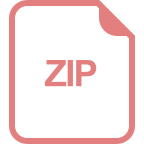
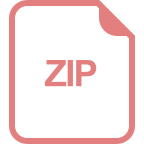
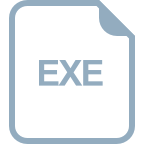
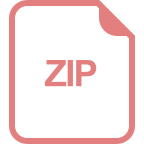
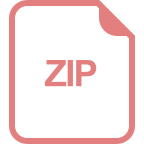
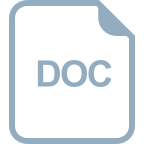
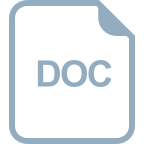
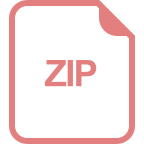
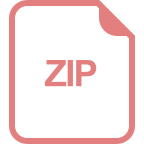
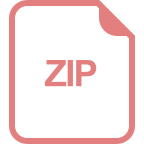
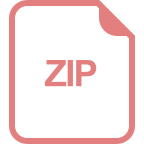
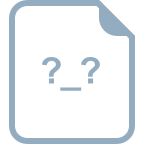
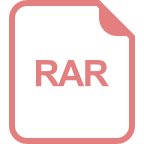
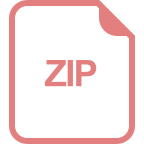
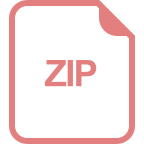
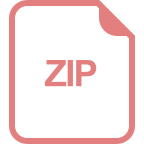
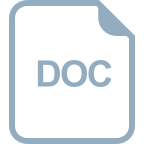
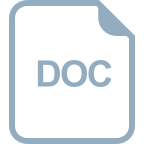