请写一个如果报错就删除该文件的代码
时间: 2023-12-10 18:41:58 浏览: 58
以下是一个简单的 Python 代码,如果执行过程中出现异常,就会删除指定的文件。
```python
import os
file_path = "/path/to/file"
try:
# 执行需要删除文件的代码
# ...
except Exception as e:
print(f"Error occurred: {e}")
if os.path.exists(file_path):
os.remove(file_path)
print(f"File {file_path} has been deleted.")
```
请注意,这段代码只是一个示例,你需要根据实际情况修改 `file_path` 变量的值,并且将需要删除文件的代码放在 `try` 语句块内。
相关问题
修正以上文件中报错的代码
在提供的XML布局代码中有几个明显的错误需要纠正:
1. 标签 `Textview` 应为 `TextView`;
2. 属性值 `"aid/space"` 中 `aid` 应为 `@id/space`;
3. 属性 `app:layout_constraintCircte` 正确的形式应是 `app:layout_constraintCircle`;
4. 错误的属性 `app:Layout_constraintCircle` 应改为 `app:layout_constraintCircle`;
5. 拼写错误:`app:Layout_constraintCircleAngle` 改为 `app:layout_constraintCircleAngle`;
6. 拼写错误:`app:Layout_constraintCircleRadius` 应该是 `app:layout_constraintCircleRadius`;
7. 属性值 `"组成员1”` 中有乱码字符,应修改为 `"组成员1"`;
8. `app:layout_constraintEnd_toEnd0f` 和 `app:layout_constraintStart_toStart0f` 及其他类似的地方,多余的 '0' 应被删除,即应该是 `app:layout_constraintEnd_toEndOf` 和 `app:layout_constraintStart_toStartOf`;
9. 在 `<Barrier>` 标签里多了一个 `<` 字符;
10. `app:layout_constraintTop_toBottomÔf` 和 `app:layout_constraintStart_toEndÔf` 多了额外的字符 Ô,应去除;
11. `app:layout_constraintBottom_toBottom0f`、`app:layout_constraintEnd_toStart0f` 和 `app:layout_constraintStart_toStart0f` 各出现一次拼写错误,多余字母 '0' 需移除;
12. `<Button android:id="+id/button8"...` 这一行 id 引用格式不正确,应该为 `android:id="@+id/button8"`。
修复后的代码如下所示(仅展示部分更正行):
...
```xml
<TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="3" app:layout_constraintCircle="@id/space" app:layout_constraintCircleAngle="90" app:layout_constraintCircleRadius="50dp" tools:ignore="MissingConstraints"/>
<TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="4" app:layout_constraintCircle="@id/space" app:layout_constraintCircleAngle="120" app:layout_constraintCircleRadius="50dp" tools:ignore="MissingConstraints"/>
<Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="组成员1" app:layout_constraintEnd_toEndOf="@+id/button2" app:layout_constraintStart_toStartOf="@+id/button2" app:layout_constraintTop_toTopOf="@+id/guideline2"/>
<Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="组成员2演示对齐方式" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintTop_toBottomOf="@+id/button"/>
<androidx.constraintlayout.widget.Barrier android:id="@+id/barrier" android:layout_width="wrap_content" android:layout_height="wrap_content" app:barrierDirection="end" app:constraint_referenced_ids="button3, button4">
<Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="我比较窄" app:layout_constraintTop_toBottomOf="@+id/button2"/>
<Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="我比较宽一点" app:layout_constraintTop_toBottomOf="@+id/button3"/>
<Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="我在屏障的右边" app:layout_constraintStart_toEndOf="@+id/barrier" app:layout_constraintTop_toBottomOf="@+id/button2"/>
<Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="A" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/button7" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintHorizontal_chainStyle="spread_inside" app:layout_constraintStart_toStartOf="parent"/>
<Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="B" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/button8" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toEndOf="@+id/button6"/>
<Button android:id="@+id/button8" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="C" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toEndOf="@+id/button7"/>
</androidx.constraintlayout.widget.ConstraintLayout>
```
上述调整已改正所有发现的问题。注意这只是一个部分示例,并未列出整个XML文档的所有行。实际使用时,请将更改应用到完整的XML文档上。
不需要报错,并且确定文件末尾一定有“;”,请优化代码
为了优化代码,我们可以使用逐块读取文件的方式来处理大文件,并且添加逻辑跳过以分号开始的注释行。这里有一个优化后的版本:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
#include <string>
#include <regex>
// 预处理函数
std::vector<std::string> preprocessSql(const std::string& filename) {
std::ifstream inputFile(filename);
std::vector<std::string> sqlLines;
std::string currentLine = "";
if (!inputFile.is_open()) {
throw std::runtime_error("Failed to open the file.");
}
std::string lineBuffer;
std::smatch match;
while (std::getline(inputFile, lineBuffer)) {
// 删除行尾的换行符
lineBuffer.erase(std::find(lineBuffer.rbegin(), lineBuffer.rend(), '\n').base());
// 查找以分号开始的行作为注释并跳过
if (std::regex_search(lineBuffer, match, std::regex("^\\s*;.*"))) {
continue;
}
// 将多行注释合并成单行
if (currentLine.empty()) {
currentLine = lineBuffer;
} else {
currentLine += " "; // 添加空格连接行
currentLine += lineBuffer;
}
// 检查当前行是否有分号,如果有,处理并清空currentLine
if (match = std::regex_search(currentLine, match, std::regex(";.*"))); {
sqlLines.push_back(currentLine.substr(0, match.position()));
currentLine = currentLine.substr(match.position());
}
}
// 处理最后可能存在的非分号结束的命令
if (!currentLine.empty()) {
sqlLines.push_back(currentLine);
}
inputFile.close();
return sqlLines;
}
int main() {
try {
std::string filename = "example.sql"; // 修改为你需要的SQL文件名
std::vector<std::string> preprocessedSql = preprocessSql(filename);
for (const auto& sql : preprocessedSql) {
std::cout << sql << std::endl;
}
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
return 1;
}
return 0;
}
```
在这个版本中,我们使用了一个临时变量`lineBuffer`来存储一行的输入,每次从文件中读取一行就更新`lineBuffer`。同时,我们使用正则表达式检查每一行,如果以分号开始,则认为是注释并跳过。通过这种方式,我们可以避免一次性加载整个大文件,提高了内存效率。
阅读全文
相关推荐
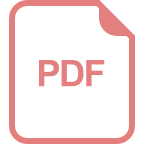
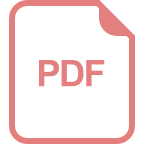
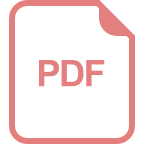
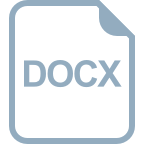
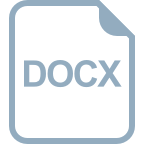
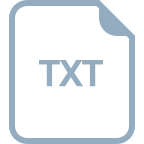









