基于tensorflow2.5,使用随机池化和L2池化替换CBAM注意力机制模块里所有的池化操作,并给出使用示例
时间: 2024-05-03 12:20:03 浏览: 22
首先,需要先导入必要的库和模块:
```python
import tensorflow as tf
from tensorflow.keras.layers import Conv2D, Dense, Flatten, MaxPooling2D, GlobalAveragePooling2D, Reshape, multiply, Add, Lambda, AveragePooling2D
```
CBAM注意力机制模块包含两个部分:Channel Attention和Spatial Attention。
对于Channel Attention,原始实现中使用了全局平均池化和全连接层,将通道维度的信息压缩到一个标量上,然后使用sigmoid激活函数进行缩放。我们可以使用随机池化或L2池化来代替全局平均池化。
对于Spatial Attention,原始实现中使用了max pooling和average pooling,分别计算通道维度上的最大值和平均值,然后将它们拼接起来,使用全连接层进行学习,最后使用sigmoid激活函数进行缩放。我们可以使用随机池化或L2池化来代替max pooling和average pooling。
下面是使用随机池化和L2池化替换CBAM注意力机制模块里所有的池化操作的示例代码:
```python
class ChannelAttention(tf.keras.layers.Layer):
def __init__(self, reduction=16):
super(ChannelAttention, self).__init__()
self.reduction = reduction
self.random_pool = Lambda(lambda x: tf.reduce_max(x, axis=[1, 2], keepdims=True))
self.l2_pool = Lambda(lambda x: tf.sqrt(tf.reduce_mean(tf.square(x), axis=[1, 2], keepdims=True) + 1e-8))
self.fc1 = Dense(units=self.reduction, activation='relu', use_bias=True)
self.fc2 = Dense(units=self.reduction, activation='relu', use_bias=True)
self.fc3 = Dense(units=1, activation='sigmoid', use_bias=True)
def call(self, inputs):
x = inputs
# Random pooling
x_random = self.random_pool(x)
# L2 pooling
x_l2 = self.l2_pool(x)
x_random = self.fc1(x_random)
x_random = self.fc2(x_random)
x_l2 = self.fc1(x_l2)
x_l2 = self.fc2(x_l2)
x_random = self.fc3(x_random)
x_l2 = self.fc3(x_l2)
scale = Add()([x_random, x_l2])
scale = multiply([inputs, scale])
return scale
class SpatialAttention(tf.keras.layers.Layer):
def __init__(self):
super(SpatialAttention, self).__init__()
self.random_pool = Lambda(lambda x: tf.image.random_crop(x, size=[tf.shape(x)[0], 1, 1, tf.shape(x)[-1]]))
self.l2_pool = Lambda(lambda x: tf.sqrt(tf.reduce_mean(tf.square(x), axis=-1, keepdims=True) + 1e-8))
self.conv1 = Conv2D(filters=1, kernel_size=7, strides=1, padding='same', use_bias=False)
self.sigmoid = Lambda(lambda x: tf.sigmoid(x))
def call(self, inputs):
x = inputs
# Random pooling
x_random = self.random_pool(x)
# L2 pooling
x_l2 = self.l2_pool(x)
x_random = self.conv1(x_random)
x_l2 = self.conv1(x_l2)
x_random = self.sigmoid(x_random)
x_l2 = self.sigmoid(x_l2)
attention = multiply([x, x_random + x_l2])
return attention
class CBAM(tf.keras.layers.Layer):
def __init__(self, reduction=16):
super(CBAM, self).__init__()
self.channel_attention = ChannelAttention(reduction)
self.spatial_attention = SpatialAttention()
def call(self, inputs):
x = inputs
x_channel = self.channel_attention(x)
x_spatial = self.spatial_attention(x)
x = multiply([x_channel, x_spatial])
return x
```
使用示例:
```python
inputs = tf.keras.Input(shape=(224, 224, 3))
x = Conv2D(filters=64, kernel_size=3, strides=1, padding='same', use_bias=False)(inputs)
x = CBAM(reduction=16)(x)
x = GlobalAveragePooling2D()(x)
outputs = Dense(units=10, activation='softmax')(x)
model = tf.keras.Model(inputs, outputs)
model.summary()
```
相关推荐
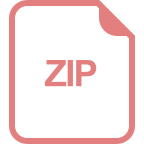














