遍历clang的ast树的完整代码
时间: 2024-05-04 08:19:11 浏览: 24
以下是遍历clang的AST树的完整代码示例:
```
#include "clang/AST/ASTConsumer.h"
#include "clang/AST/ASTContext.h"
#include "clang/AST/Decl.h"
#include "clang/AST/DeclGroup.h"
#include "clang/AST/RecursiveASTVisitor.h"
#include "clang/Frontend/CompilerInstance.h"
#include "llvm/Support/raw_ostream.h"
using namespace clang;
class MyASTVisitor : public RecursiveASTVisitor<MyASTVisitor> {
public:
bool VisitFunctionDecl(FunctionDecl *func) {
llvm::outs() << "Visited function: " << func->getNameAsString() << "\n";
return true;
}
};
class MyASTConsumer : public ASTConsumer {
public:
virtual bool HandleTopLevelDecl(DeclGroupRef DG) {
for (DeclGroupRef::iterator i = DG.begin(), e = DG.end(); i != e; ++i) {
Visitor.TraverseDecl(*i);
}
return true;
}
private:
MyASTVisitor Visitor;
};
class MyFrontendAction : public ASTFrontendAction {
public:
virtual std::unique_ptr<ASTConsumer> CreateASTConsumer(CompilerInstance &CI,
StringRef file) {
return std::make_unique<MyASTConsumer>();
}
};
int main(int argc, const char **argv) {
if (argc > 1) {
clang::tooling::runToolOnCode(std::make_unique<MyFrontendAction>(), argv[1]);
}
return 0;
}
```
此代码使用了clang的ASTConsumer、RecursiveASTVisitor和ASTFrontendAction类,它们可以让我们访问和遍历clang的AST树。
MyASTVisitor是我们自己定义的类,继承于RecursiveASTVisitor类,并覆写了VisitFunctionDecl方法。在这个例子中,我们只是简单地输出了访问到的每个函数声明的名称。如果你需要遍历不同类型的声明或表达式,可以在MyASTVisitor中添加其他Visit*方法。
MyASTConsumer类是ASTConsumer的子类,用于处理顶层声明。在HandleTopLevelDecl方法中,我们通过调用Visitor.TraverseDecl遍历了每个顶级声明。TraverseDecl方法会调用MyASTVisitor中对应的Visit*方法,从而遍历整个AST树。
MyFrontendAction类是ASTFrontendAction的子类,用于创建ASTConsumer。在CreateASTConsumer方法中,我们返回了MyASTConsumer的实例。
最后,在main函数中,我们使用clang::tooling::runToolOnCode方法运行了这个工具。它会读取我们传递的代码字符串,并调用MyFrontendAction来创建ASTConsumer,从而遍历整个AST树。
需要注意的是,这里只是一个简单的示例,如果你需要遍历更复杂的AST树,可能需要添加更多的Visit*方法和处理代码。
相关推荐
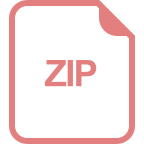
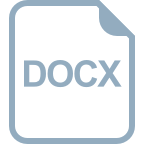
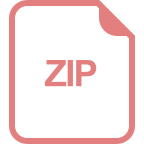










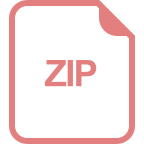
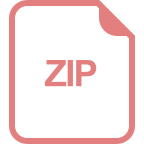
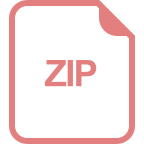
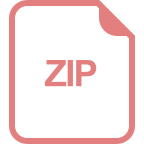
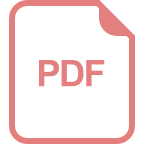