qt读入矩阵数据
时间: 2023-07-13 19:10:21 浏览: 128
可以使用QFile和QTextStream将txt文件中的矩阵数据读入到QVector或QList中。以下是一个示例代码:
```c++
QList<QVector<double>> matrixData;
QFile file("matrix.txt");
if (file.open(QIODevice::ReadOnly | QIODevice::Text)) {
QTextStream stream(&file);
while (!stream.atEnd()) {
QString line = stream.readLine();
QVector<double> row;
QStringList values = line.split(" ");
for (QString value : values) {
row.append(value.toDouble());
}
matrixData.append(row);
}
file.close();
}
```
这段代码会读取matrix.txt文件中的每一行数据,将其转换为QVector<double>类型的一行,并将每一行添加到QList<QVector<double>>类型的matrixData变量中。你可以根据实际情况修改代码中的文件名和变量类型。注意,在上述示例中,矩阵中的数据以空格分隔,如果你的txt文件采用其他分隔符,需要相应地修改代码。
相关问题
qt读入声音转化为数组
在Qt中,你可以使用QAudioInput或QMediaPlayer等类来处理音频输入,并将声音数据转换为数组。以下是一个简单的步骤概述:
1. **包含必要的库**:
首先,在你的`.pro`文件或者源代码中,确保包含了`QtCore`和`QtMultimedia`库。
```cpp
QT += multimedia multimediawidgets
```
2. **设置音频输入**:
使用`QAudioInput`类创建一个音频输入对象,并打开设备:
```cpp
QAudioFormat format;
format.setSampleRate(44100); // 采样率,可根据需要调整
format.setChannelCount(1); // 单声道
QAudioInput audioInput(format);
if (!audioInput.open(QIODevice::ReadOnly)) {
qDebug() << "Failed to open audio input";
}
```
3. **获取声音数据**:
调用`read()`函数获取一小块音频数据,将其存储到缓冲区中。这通常会返回一个`QByteArray`或`char*`类型的数组:
```cpp
QByteArray audioBuffer;
while (audioInput.read(audioBuffer) == QAudioBuffer::Ok) {
// 将读取的数据转换成合适的数组类型,比如float* 或 qint16*
float* audioArray = static_cast<float*>(audioBuffer.data());
// 这里可以进一步处理音频数据,如处理、分析等
}
```
4. **关闭资源**:
当不再需要音频输入时,记得关闭它:
```cpp
audioInput.close();
```
c++qt如何输出矩阵
在C++中使用Qt框架输出矩阵可以通过多种方式实现。以下是使用Qt进行矩阵输出的一种常见方法:
1. 创建一个矩阵模型,可以使用`QList`或者其他容器来存储矩阵数据。
2. 使用`QTableView`或者自定义的`QWidget`来显示矩阵。
3. 利用`QStandardItemModel`来构建数据模型,然后将这个模型设置给`QTableView`。
以下是一个简单的示例代码,展示如何创建一个简单的矩阵并将其输出到控制台:
```cpp
#include <QCoreApplication>
#include <QList>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 定义一个矩阵,这里以3x3的整数矩阵为例
QList<QList<int>> matrix;
matrix.append(QList<int>() << 1 << 2 << 3);
matrix.append(QList<int>() << 4 << 5 << 6);
matrix.append(QList<int>() << 7 << 8 << 9);
// 遍历矩阵并输出到控制台
for (int i = 0; i < matrix.size(); ++i) {
for (int j = 0; j < matrix[i].size(); ++j) {
qDebug() << matrix[i][j];
}
}
return a.exec();
}
```
这段代码首先定义了一个3x3的整数矩阵,并使用`QList`的嵌套来实现。然后通过两层嵌套的循环遍历这个矩阵,并使用`qDebug()`函数输出矩阵中的每个元素。
在实际的Qt应用程序中,如果你需要在图形界面中显示矩阵,可以使用`QTableView`控件,并通过`QStandardItemModel`来填充数据。
阅读全文
相关推荐
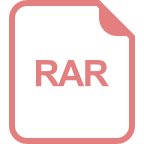
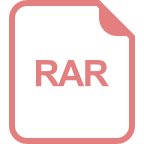
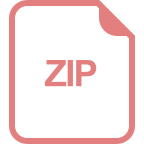













