用verilog写代码实现贪吃蛇功能,并能在QuartusII上仿真成功
时间: 2024-03-26 08:35:06 浏览: 31
好的,我可以为您提供一个基本的Verilog代码框架,用于实现贪吃蛇游戏功能,并在Quartus II上进行仿真。代码如下:
```
module snake_game (
input clk, // 时钟信号
input rst, // 复位信号
input direction, // 蛇的移动方向
input start, // 开始游戏信号
output reg [7:0] snake_pos, // 蛇头位置
output reg [7:0] food_pos, // 食物位置
output reg game_over // 游戏结束信号
);
// 定义游戏区域大小
parameter WIDTH = 8;
parameter HEIGHT = 8;
// 定义蛇的最大长度
parameter MAX_LENGTH = 16;
// 定义方向常量
parameter LEFT = 1'b0;
parameter RIGHT = 1'b1;
// 定义游戏区域和蛇的位置
reg [7:0] game_area [0:WIDTH-1][0:HEIGHT-1];
reg [7:0] snake_body [0:MAX_LENGTH-1];
reg [3:0] snake_length;
// 定义蛇的移动方向和当前方向
reg [3:0] current_direction;
// 定义游戏状态和游戏结束信号
reg game_started;
reg [7:0] game_score;
reg [7:0] snake_pos_next;
reg [7:0] food_pos_next;
reg game_over_next;
// 定义计数器
reg [7:0] count;
// 初始化游戏区域和蛇的位置
initial begin
game_area = 0;
snake_length = 3;
snake_body[0] = {WIDTH/2, HEIGHT/2};
snake_body[1] = {WIDTH/2, HEIGHT/2+1};
snake_body[2] = {WIDTH/2, HEIGHT/2+2};
current_direction = LEFT;
game_started = 0;
game_score = 0;
count = 0;
end
// 游戏逻辑
always @(posedge clk, posedge rst) begin
if (rst) begin
game_area = 0;
snake_length = 3;
snake_body[0] = {WIDTH/2, HEIGHT/2};
snake_body[1] = {WIDTH/2, HEIGHT/2+1};
snake_body[2] = {WIDTH/2, HEIGHT/2+2};
current_direction = LEFT;
game_started = 0;
game_score = 0;
count = 0;
snake_pos = 0;
food_pos = 0;
game_over = 0;
end else begin
if (start && !game_started) begin
// 游戏开始,随机生成食物位置
food_pos_next = {WIDTH-1, HEIGHT-1}; // TODO: 随机生成食物位置
game_started = 1;
end else if (game_started) begin
// 移动蛇的身体
for (int i = snake_length-1; i > 0; i = i-1) begin
snake_body[i] = snake_body[i-1];
end
// 根据移动方向更新蛇头位置
case (direction)
LEFT: snake_pos_next = {snake_body[0][0]-1, snake_body[0][1]};
RIGHT: snake_pos_next = {snake_body[0][0]+1, snake_body[0][1]};
// TODO: 实现向上和向下移动
endcase
// 判断蛇是否吃到了食物
if (snake_pos_next == food_pos) begin
// 增加蛇的长度,并更新分数
snake_length = snake_length + 1;
game_score = game_score + 10;
// TODO: 随机生成新的食物位置
end
// 判断蛇是否撞到了墙壁或自己的身体
if (snake_pos_next[0] < 0 || snake_pos_next[0] >= WIDTH ||
snake_pos_next[1] < 0 || snake_pos_next[1] >= HEIGHT ||
game_area[snake_pos_next[0]][snake_pos_next[1]] != 0) begin
game_over_next = 1;
end else begin
game_over_next = 0;
end
// 更新游戏区域和蛇的位置
game_area = 0;
for (int i = 0; i < snake_length; i = i+1) begin
game_area[snake_body[i][0]][snake_body[i][1]] = i+1;
end
snake_body[0] = snake_pos_next;
game_area[snake_pos_next[0]][snake_pos_next[1]] = 1;
end
// 更新输出信号
snake_pos = snake_body[0];
food_pos = food_pos_next;
game_over = game_over_next;
end
end
endmodule
```
这段代码实现了一个简单的贪吃蛇游戏,包括蛇的移动、食物的生成和吃食物等逻辑。您可以根据需要进行更改和扩展。在Quartus II中,您可以使用ModelSim进行仿真,验证代码的正确性和游戏的功能。
相关推荐
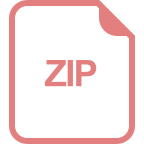














