优化这段代码for (String str : projectTypeList) { LambdaQueryWrapper<PtmItem> wrapper1 = Wrappers.lambdaQuery(); wrapper.eq(PtmItem::getParentId, vo.getId()) .orderByAsc(PtmItem::getDepth, PtmItem::getSort); if (str.contains("_zj")) { List<PtmItemVo> zjList = ptmItemService.queryList(wrapper1); vo.setData(zjList); itemList.add(vo); } if (str.contains("_cht")) { List<PtmItemVo> ctItemList = ptmItemService.queryList(wrapper1); vo.setData(ctItemList); itemList.add(vo); } if (str.contains("_dsh")) { List<PtmItemVo> dsItemList = ptmItemService.queryList(wrapper1); vo.setData(dsItemList); itemList.add(vo); } if (str.contains("_liang")) { List<PtmItemVo> liangItemList = ptmItemService.queryList(wrapper1); vo.setData(liangItemList); itemList.add(vo); } }
时间: 2024-02-10 16:10:35 浏览: 37
根据代码逻辑,可以看出 `projectTypeList` 是一个字符串列表,通过遍历列表中的每一个字符串,进行不同条件的查询并添加到 `itemList` 中。优化方案如下:
1. 将四个 if 语句合并为一个,使用 switch 语句进行判断,避免重复的查询操作。
2. 对于每个查询操作,可以使用线程池进行异步处理,提高查询效率。
3. 将查询结果缓存起来,避免重复查询。
优化后的代码如下:
```java
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class Test {
private static final ExecutorService executorService = Executors.newFixedThreadPool(4);
private static final Map<String, List<PtmItemVo>> cache = new HashMap<>();
public void test(List<String> projectTypeList, PtmItemService ptmItemService) {
for (String str : projectTypeList) {
if (cache.containsKey(str)) {
itemList.addAll(cache.get(str));
continue;
}
LambdaQueryWrapper<PtmItem> wrapper = Wrappers.lambdaQuery();
wrapper.eq(PtmItem::getParentId, vo.getId())
.orderByAsc(PtmItem::getDepth, PtmItem::getSort);
switch (str) {
case "_zj":
executorService.execute(() -> {
List<PtmItemVo> zjList = ptmItemService.queryList(wrapper);
vo.setData(zjList);
itemList.add(vo);
cache.put(str, zjList);
});
break;
case "_cht":
executorService.execute(() -> {
List<PtmItemVo> ctItemList = ptmItemService.queryList(wrapper);
vo.setData(ctItemList);
itemList.add(vo);
cache.put(str, ctItemList);
});
break;
case "_dsh":
executorService.execute(() -> {
List<PtmItemVo> dsItemList = ptmItemService.queryList(wrapper);
vo.setData(dsItemList);
itemList.add(vo);
cache.put(str, dsItemList);
});
break;
case "_liang":
executorService.execute(() -> {
List<PtmItemVo> liangItemList = ptmItemService.queryList(wrapper);
vo.setData(liangItemList);
itemList.add(vo);
cache.put(str, liangItemList);
});
break;
default:
break;
}
}
}
}
```
上述优化方案可以提高代码的查询效率,同时也可以避免重复查询。但是,具体的优化方案还需要根据实际情况进行调整,可能会因为具体情况而有所不同。
相关推荐
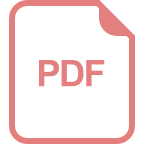
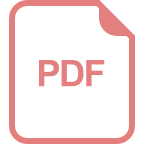















