用C++写一个·图书管理系统
时间: 2024-09-23 14:05:55 浏览: 46
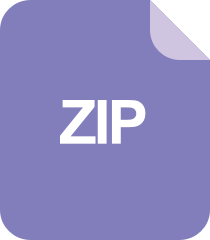
【C++课程设计】图书管理系统

在C++中编写一个简单的图书管理系统涉及到几个关键部分,包括数据结构、文件操作以及用户界面交互。这里是一个基础的控制台版图书管理系统的简化示例:
```cpp
#include <iostream>
#include <map>
#include <fstream>
// 定义图书结构体
struct Book {
std::string title;
std::string author;
int stock;
};
class Library {
private:
std::map<std::string, Book> books; // 使用关联容器存储书籍信息
public:
// 添加新书
void addBook(const std::string& title, const std::string& author, int stock) {
books[title] = {title, author, stock};
}
// 查询书籍库存
bool hasStock(const std::string& title) {
return books.find(title) != books.end() && books[title].stock > 0;
}
// 出借书籍
bool borrowBook(const std::string& title) {
if (hasStock(title)) {
books[title].stock--;
std::cout << "Borrowed: " << title << "\n";
return true;
}
std::cout << "Sorry, out of stock.\n";
return false;
}
// 归还书籍并检查库存
bool returnBook(const std::string& title) {
if (books.find(title) != books.end()) {
books[title].stock++;
std::cout << "Returned: " << title << "\n";
return true;
}
std::cout << "Invalid book to return.\n";
return false;
}
};
int main() {
Library lib;
// 示例操作
lib.addBook("The Catcher in the Rye", "J.D. Salinger", 5);
lib.borrowBook("The Catcher in the Rye");
lib.returnBook("The Catcher in the Rye");
// 文件持久化(这里仅作演示,实际应用会更复杂)
try {
std::ofstream file("library.txt");
for (const auto& entry : books) {
file << entry.first << ": " << entry.second.title << ", " << entry.second.author << ", " << entry.second.stock << '\n';
}
file.close();
} catch (std::exception& e) {
std::cerr << "Error writing to file: " << e.what() << '\n';
}
return 0;
}
```
这个程序定义了一个`Library`类,包含了添加、查询库存、出借和归还书籍的功能。它还展示了如何将数据保存到磁盘以便持久化。注意这只是一个基础版本,实际应用可能需要更复杂的错误处理、用户输入验证以及图形用户界面。
阅读全文
相关推荐
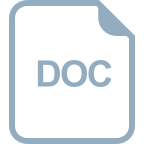
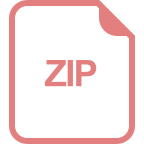
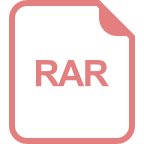
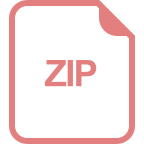
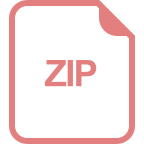
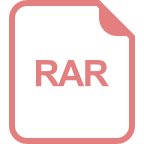
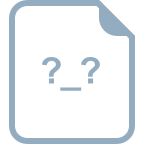
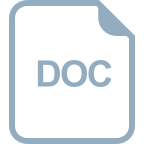
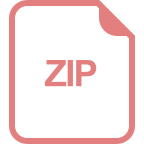
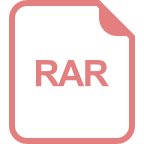
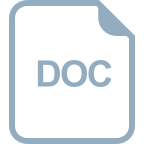
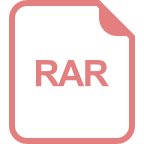
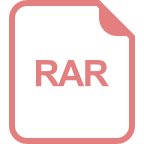
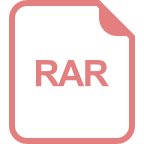