题目为: min [𝑧=𝑥_1^2+𝑥_2^2+3𝑥_3^2+4𝑥_4^2+2𝑥_5^2−8𝑥_1−2𝑥_2−2𝑥_3−𝑥_4−2𝑥_5 ] 𝑠.𝑡.{ 0 <= x_i <= 99,且x_i 为整数,i =1,2,3,...,5, 𝑥_1+𝑥_2 +𝑥_3 +𝑥_4 +𝑥_5≤400, 𝑥_1+2𝑥_2 +2𝑥_3 +𝑥_4 +6𝑥_5≤800, 2𝑥_1+𝑥_2 +6𝑥_3 <= 200, 𝑥_3+𝑥_4 +5𝑥_5≤200 } 用matlab语言实现遗传算法求解非线性规划问题(不用ga函数)
时间: 2024-04-18 12:26:14 浏览: 141
这个非线性规划问题可以使用遗传算法来求解。下面是用MATLAB语言实现遗传算法的代码:
```matlab
% 参数设置
popSize = 100; % 种群大小
chromLength = 5; % 染色体长度
maxGen = 100; % 最大迭代次数
pc = 0.8; % 交叉概率
pm = 0.01; % 变异概率
% 初始化种群
population = randi([0, 99], popSize, chromLength);
% 迭代寻优
for gen = 1:maxGen
% 计算适应度值
fitness = evaluateFitness(population);
% 选择操作(轮盘赌选择)
selectedPopulation = selection(population, fitness);
% 交叉操作
offspringPopulation = crossover(selectedPopulation, pc);
% 变异操作
mutatedPopulation = mutation(offspringPopulation, pm);
% 合并父代和子代种群
population = [population; mutatedPopulation];
% 保留最优个体
[~, idx] = sort(evaluateFitness(population), 'descend');
population = population(idx(1:popSize), :);
end
% 输出结果
bestIndividual = population(1, :);
bestFitness = evaluateFitness(bestIndividual);
disp(['最优解:', num2str(bestIndividual)]);
disp(['最优适应度值:', num2str(bestFitness)]);
% 计算适应度值的函数
function fitness = evaluateFitness(population)
[n, ~] = size(population);
fitness = zeros(n, 1);
for i = 1:n
x = population(i, :);
fitness(i) = x(1)^2 + x(2)^2 + 3*x(3)^2 + 4*x(4)^2 + 2*x(5)^2 - 8*x(1) - 2*x(2) - 2*x(3) - x(4) - 2*x(5);
end
end
% 轮盘赌选择操作
function selectedPopulation = selection(population, fitness)
[n, ~] = size(population);
totalFitness = sum(fitness);
probability = fitness / totalFitness;
cumulativeProbability = cumsum(probability);
selectedPopulation = zeros(n, size(population, 2));
for i = 1:n
r = rand();
idx = find(cumulativeProbability >= r, 1);
selectedPopulation(i, :) = population(idx, :);
end
end
% 交叉操作
function offspringPopulation = crossover(selectedPopulation, pc)
[n, chromLength] = size(selectedPopulation);
offspringPopulation = zeros(n, chromLength);
for i = 1:2:n-1
if rand() < pc
crossoverPoint = randi([2, chromLength-1]);
offspringPopulation(i, :) = [selectedPopulation(i, 1:crossoverPoint), selectedPopulation(i+1, crossoverPoint+1:end)];
offspringPopulation(i+1, :) = [selectedPopulation(i+1, 1:crossoverPoint), selectedPopulation(i, crossoverPoint+1:end)];
else
offspringPopulation(i, :) = selectedPopulation(i, :);
offspringPopulation(i+1, :) = selectedPopulation(i+1, :);
end
end
end
% 变异操作
function mutatedPopulation = mutation(offspringPopulation, pm)
[n, chromLength] = size(offspringPopulation);
mutatedPopulation = offspringPopulation;
for i = 1:n
for j = 1:chromLength
if rand() < pm
mutatedPopulation(i, j) = randi([0, 99]);
end
end
end
end
```
上述代码实现了遗传算法的基本框架,包括初始化种群、计算适应度值、选择操作、交叉操作和变异操作等。你可以将问题中的目标函数和约束条件替换到代码中的相应部分,然后运行代码即可求解该非线性规划问题。
阅读全文
相关推荐
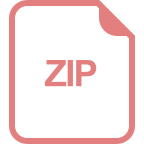
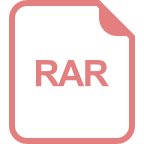
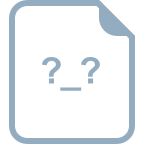
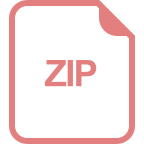
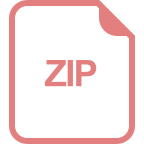
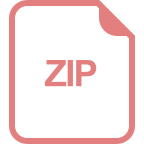
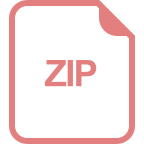
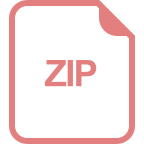
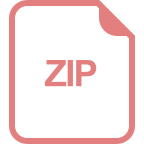
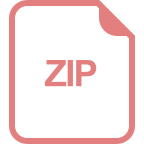
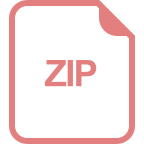
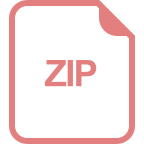
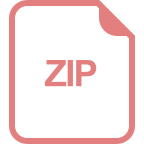
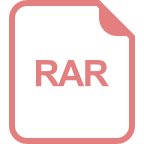
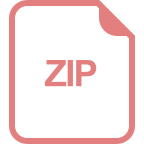
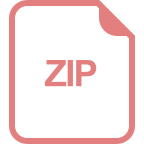