数据结构C语言两个矩阵先压缩再相加的完整代码
时间: 2024-10-21 20:11:01 浏览: 24
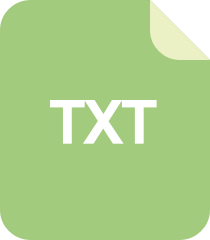
两个数相加c语言
在C语言中,为了压缩两个矩阵并进行相加操作,首先需要考虑的是矩阵是否可以被压缩,即行数和列数都是一样的,并且非零元素很少。如果满足这个条件,可以采用稀疏矩阵数据结构(如邻接列表或三元组表示法)来存储。然而,由于C语言的标准库并不直接支持稀疏矩阵处理,我们需要自定义实现。
这里给出一个简单的示例,假设我们有一个稀疏矩阵的简单表示,其中`sparse_matrix`是一个结构体,包含行、列和值。然后我们可以创建两个这样的结构体数组,分别代表两个矩阵。相加操作仅对非零元素进行:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct sparse_matrix {
int row;
int col;
double value;
} SparseMatrix;
SparseMatrix** compress_and_add(SparseMatrix* mat1, SparseMatrix* mat2) {
// 假设已经检查过两个矩阵的维度和非零元素一致
int rows = mat1->row, cols = mat1->col;
SparseMatrix* result[rows][cols]; // 初始化结果矩阵
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
if (mat1[i].value != 0 && mat2[i].value != 0) {
result[i][j] = malloc(sizeof(SparseMatrix));
result[i][j]->row = i;
result[i][j]->col = j;
result[i][j]->value = mat1[i].value + mat2[i].value;
}
}
}
return result;
}
void free_sparse_matrix(SparseMatrix** matrix, int rows, int cols) {
for (int i = 0; i < rows; ++i)
for (int j = 0; j < cols; ++j)
if (matrix[i][j])
free(matrix[i][j]);
free(matrix);
}
// 示例用法
int main() {
// 创建示例矩阵,实际应用需从文件或其他来源读取
SparseMatrix mat1[] = { {0, 0, 4}, {1, 1, 2}, {2, 2, 6} };
SparseMatrix mat2[] = { {0, 1, 1}, {1, 0, 3}, {2, 2, 5} };
int rows = sizeof(mat1) / sizeof(mat1[0]), cols = sizeof(mat1[0]) / sizeof(mat1[0].value);
SparseMatrix*** compressed = compress_and_add(&mat1[0], &mat2[0]);
// 输出或保存压缩后的矩阵
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
if (compressed[i][j])
printf("(%d,%d): %.2lf\n", compressed[i][j]->row, compressed[i][j]->col, compressed[i][j]->value);
}
}
free_sparse_matrix(compressed, rows, cols);
return 0;
}
```
阅读全文
相关推荐
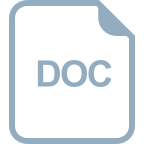
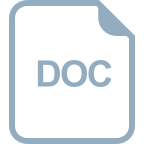
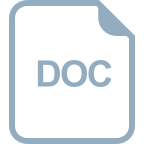
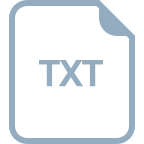
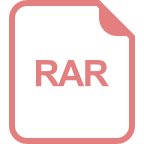
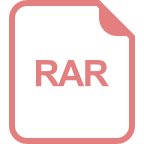
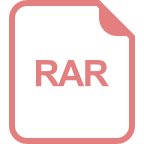
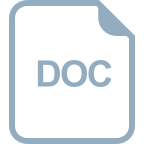
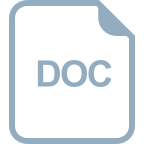
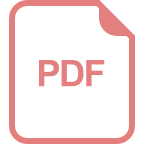
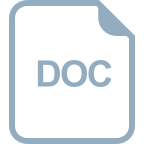
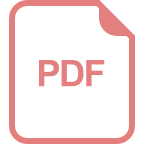
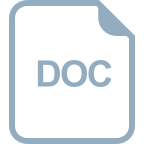
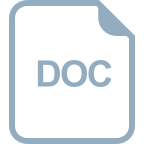
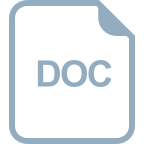
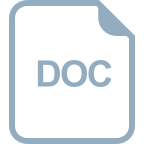
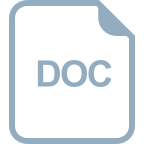
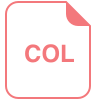