golang使用创建es索引并指定自定义的mapping 使用v8版本的api
时间: 2024-09-21 18:05:19 浏览: 43
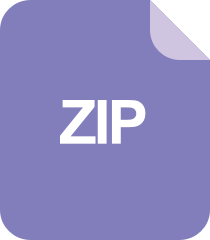
自定义api后端程序生成.zip
在Go语言中,使用Elasticsearch(ES)库,如`github.com/elastic/go-elasticsearch/v7`,可以方便地操作ES。如果你想要创建索引并指定自定义映射(Mapping),你可以按照以下步骤操作:
首先,确保安装了所需的包:
```bash
go get github.com/elastic/go-elasticsearch/v7
go get github.com/elastic/go-elasticsearch/v7/esapi
```
然后,你可以创建一个索引,并指定映射(Mapping)结构体,例如:
```go
package main
import (
"context"
"encoding/json"
"log"
"github.com/elastic/go-elasticsearch/v7"
"github.com/elastic/go-elasticsearch/v7/esapi"
)
type CustomMapping struct {
// 定义你的自定义字段及其数据类型
Field1 *string `json:"field1"`
Field2 int `json:"field2"`
}
func createIndex(client *elasticsearch.Client, indexName string, mappingCustom Mapping) error {
body, err := json.Marshal(mappingCustom)
if err != nil {
return err
}
indexRequest := esapi.IndexRequest{
Index: indexName,
DocumentID: "_doc",
Body: bytes.NewReader(body),
}
res, err := client.Do(context.Background(), indexRequest)
if err != nil {
return err
}
defer res.Body.Close()
log.Printf("Response status: %s\n", res.Status)
log.Printf("Response headers: %v\n", res.Header)
return nil
}
func main() {
// 创建Elasticsearch客户端
client, err := elasticsearch.NewClient(
elasticsearch.SetURL("http://localhost:9200"),
elasticsearch.SetBasicAuth("username", "password"), // 如果需要的话提供认证信息
)
if err != nil {
log.Fatal(err)
}
// 索引名和自定义映射
indexName := "my_index"
mappingCustom := CustomMapping{Field1: "example_value", Field2: 123}
err = createIndex(client, indexName, mappingCustom)
if err != nil {
log.Fatal(err)
}
}
```
在这个示例中,你需要将`"username"`和`"password"`替换为你实际的ES集群用户名和密码,以及`"http://localhost:9200"`替换为ES服务器地址。
阅读全文
相关推荐
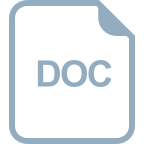
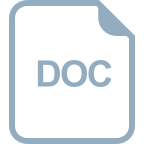
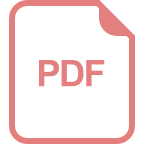
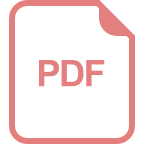
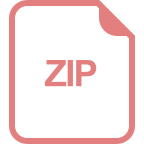
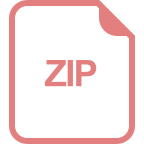
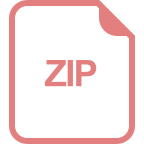
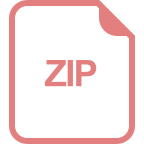
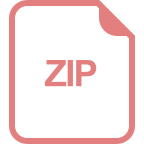
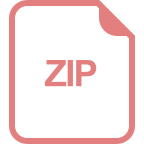
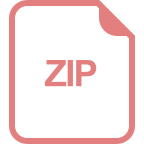
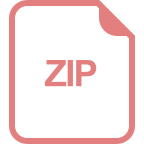
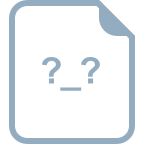
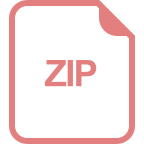
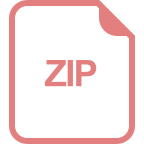
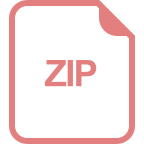
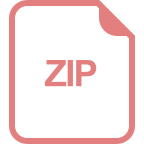