jszip解压文件出现object promise怎么解决
时间: 2024-11-15 17:20:37 浏览: 9
当你在JavaScript中使用jszip库解压缩文件并遇到"object Promise"错误时,这通常是因为jszip返回的是一个Promise对象,而你在尝试直接访问其结果而不是等待Promise解析完成。以下是解决这个问题的步骤:
1. 理解Promise:在JavaScript异步操作中,jszip函数会立即返回一个Promise,表示解压过程还未完成。你需要通过`.then()`方法处理这个Promise,当解压完成后,才能获取到实际的数据。
例如:
```javascript
const jsZip = require('jszip');
let zipFile = new JSZip(fileContent); // fileContent是你从服务器或本地获取的zip数据
zipFile.async("fileToExtract") // 假设你想解压名为"fileToExtract"的文件
.then((content) => {
// content就是解压后的文件内容,你可以在这里进行后续操作
console.log(content);
})
.catch((error) => {
console.error('Error during extraction:', error);
});
```
相关问题
JavaScript如何解决返回[object Promise]
当使用JavaScript中的Promise时,当您尝试访问Promise的值时,您可能会看到返回值为`[object Promise]`的情况。这是因为Promise是一种异步操作,它不能立即返回结果,而是需要等待操作完成后返回结果。
要访问Promise的值,您需要使用Promise的then()方法,该方法接受一个回调函数作为参数,该回调函数在Promise被解析(resolved)时被调用。例如:
```javascript
const myPromise = new Promise((resolve, reject) => {
// 执行异步操作
// 当操作完成时调用resolve()或reject()
});
myPromise.then(result => {
// 在Promise被解析时执行的回调函数
console.log(result); // 输出Promise的值
}).catch(error => {
// 在Promise被拒绝时执行的回调函数
console.error(error); // 输出Promise被拒绝的原因
});
```
在这个示例中,当Promise被解析时,then()方法中的回调函数将被调用,并且Promise的值将作为参数传递给它。如果Promise被拒绝,则catch()方法中的回调函数将被调用,并且Promise被拒绝的原因将作为参数传递给它。
请注意,您还可以使用async/await来等待Promise解析。例如:
```javascript
async function myFunction() {
const myPromise = new Promise((resolve, reject) => {
// 执行异步操作
// 当操作完成时调用resolve()或reject()
});
try {
const result = await myPromise;
console.log(result); // 输出Promise的值
} catch (error) {
console.error(error); // 输出Promise被拒绝的原因
}
}
```
在这个示例中,我们使用async函数和await操作符来等待Promise的解析。在try块中,我们等待myPromise解析,并将其值存储在result变量中。如果Promise被拒绝,则控制流将跳转到catch块中,其中我们可以处理Promise被拒绝的原因。
object Promise
Promise is an object in JavaScript that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. It is used for handling asynchronous operations such as making API calls, fetching data from a server, or reading files.
A Promise object can be in one of three states: pending, resolved, or rejected. When a promise is pending, it means the asynchronous operation is still ongoing. Once the operation is completed successfully, the promise is resolved, and if there is an error, the promise is rejected.
The typical syntax for creating a Promise object is as follows:
```javascript
const myPromise = new Promise((resolve, reject) => {
// Perform an asynchronous operation
// If successful, call resolve(value)
// If there's an error, call reject(error)
});
```
You can then use methods such as `.then()` and `.catch()` to handle the resolved value or the error respectively. The `.then()` method takes a callback function that will be executed when the promise is resolved, and the `.catch()` method handles any errors that occur during the promise's execution.
Promises provide a more structured way of dealing with asynchronous operations and make it easier to handle success and error cases. They are widely used in modern JavaScript applications.
阅读全文
相关推荐
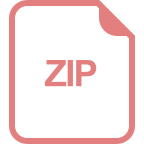
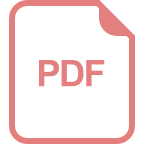
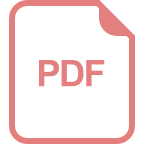













