用wxpython写程序,实现一个考试成绩查询系统 要求1.第一个界面有三个内容,第一输入学号,第二输入姓名,第三有个开始查询按钮 2.点击开始查询后,弹出学生科目与对应成绩界面,并显示总分和班级排名 3.所有学生成绩在脚本内写好,做好数据处理
时间: 2024-12-22 20:16:10 浏览: 16
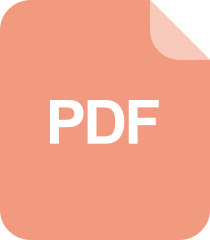
action in wxpython 中文版

在wxPython中编写一个简单的考试成绩查询系统,你可以按照以下步骤来进行:
1. 首先,你需要安装`wxPython`库,如果你还没有安装,可以使用pip进行安装:
```
pip install wxPython
```
2. 创建一个新的Python文件,例如`score_system.py`,然后引入必要的模块并创建窗口:
```python
import wx
from collections import defaultdict
# 假设这是你的考试成绩数据
student_scores = {
'001': {'张三': [85, 90, 92], '总分': 267},
# ...添加其他学生的数据
}
class ScoreQueryApp(wx.App):
def OnInit(self):
frame = QueryFrame(parent=None)
self.SetTopWindow(frame)
frame.Show()
return True
class QueryFrame(wx.Frame):
def __init__(self, parent, title='成绩查询'):
super().__init__(parent, id=wx.ID_ANY, title=title,
size=(400, 300))
self.init_ui()
def init_ui(self):
panel = wx.Panel(self)
sizer = wx.BoxSizer(wx.VERTICAL)
input_sizer = wx.FlexGridSizer(cols=2, vgap=5, hgap=10)
input_sizer.AddMany([
(wx.StaticText(panel, label="学号:"), 0, wx.ALIGN_RIGHT),
(wx.TextCtrl(panel, id=wx.ID_ANY, value='', style=wx.TE_PROCESS_ENTER),
0, wx.EXPAND),
(wx.StaticText(panel, label="姓名:"), 0, wx.ALIGN_RIGHT),
(wx.TextCtrl(panel, id=wx.ID_ANY, value='', style=wx.TE_PROCESS_ENTER),
0, wx.EXPAND),
(wx.Button(panel, label="开始查询", id=wx.ID_OK), 0, wx.EXPAND),
])
sizer.Add(input_sizer, proportion=1, flag=wx.EXPAND)
result_panel = wx.ScrolledWindow(panel)
result_sizer = wx.FlexGridSizer(rows=3, cols=2, vgap=5, hgap=10)
result_panel.SetSizer(result_sizer)
result_panel.SetupScrolling()
sizer.Add(result_panel, proportion=1, flag=wx.EXPAND | wx.ALL, border=10)
panel.SetSizer(sizer)
self.Bind(wx.EVT_TEXT_ENTER, self.on_search, id=wx.ID_ANY)
def on_search(self, event):
text_ctrl = event.GetEventObject()
if text_ctrl.GetId() == wx.ID_OK:
search_id = text_ctrl.GetValue().strip()
name = other_text_ctrl.GetValue().strip()
self.show_results(search_id, name)
def show_results(self, student_id, name):
results = student_scores.get(student_id, {})
if name and name not in results:
wx.MessageBox(f"未找到名为'{name}'的学生信息.")
return
result_sizer.Clear(True)
total_score = sum(results[name])
rank = 1
for subject, score in results[name]:
result_sizer.Add((f"{subject}: {score}", 0, wx.ALIGN_LEFT), row=rank, col=0)
rank += 1
result_sizer.Add((-1, -1), row=rank, col=0) # 添加分割线
result_sizer.Add((f"总分: {total_score}", 0, wx.ALIGN_CENTER), row=rank, col=0)
rank += 1
if len(student_scores) > 1:
sorted_scores = sorted(student_scores.items(), key=lambda x: x[1]['总分'], reverse=True)
for i, (id, _) in enumerate(sorted_scores):
if id == student_id:
break
rank += 1
result_sizer.Add((f"班级排名: {i+1}/{len(sorted_scores)}", 0, wx.ALIGN_LEFT), row=rank, col=0)
result_panel.Layout()
result_panel.Update()
if __name__ == "__main__":
app = ScoreQueryApp()
app.MainLoop()
```
这个示例包含了基本的用户界面和搜索功能,但为了实际运行,你需要将上面的`student_scores`字典替换为你的真实成绩数据。当你运行此程序时,它会启动一个窗口,让你输入学号和姓名进行查询。
阅读全文
相关推荐
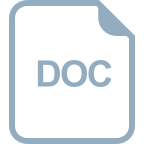
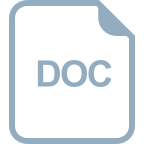
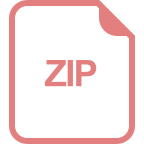
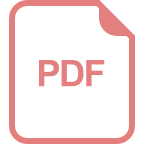
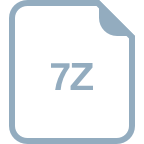
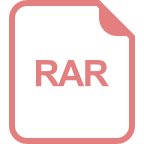
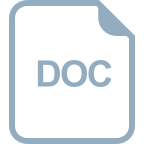
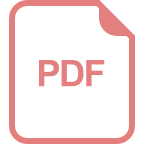
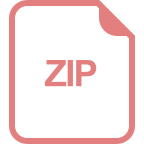
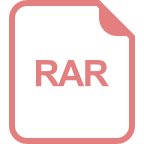




