快速排序算法c++实现
时间: 2024-10-28 22:04:11 浏览: 10
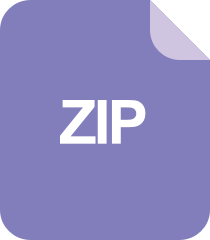
快速排序算法C++实现(超详细解析)
快速排序是一种高效的排序算法,它基于分治策略,通过一趟排序将待排记录分隔成独立的两部分,其中一部分的所有数据都比另一部分的所有数据都要小,然后对这两部分继续进行快速排序,直到整个序列有序。
以下是快速排序的一个基本的C++实现:
```cpp
#include <iostream>
using namespace std;
// 交换两个元素的位置
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
// 分割函数,选择第一个元素作为基准值
int partition(int arr[], int low, int high) {
int pivot = arr[low]; // 基准值
while (low < high) {
while (low < high && arr[high] >= pivot) high--; // 将大于等于pivot的元素移到左边
if (low < high) swap(&arr[low], &arr[high]);
while (low < high && arr[low] <= pivot) low++; // 将小于等于pivot的元素移到右边
if (low < high) swap(&arr[low], &arr[high]);
}
swap(&arr[low], &arr[high]); // 将基准值放到最终位置
return low;
}
// 快速排序主函数
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1); // 对左半部分递归排序
quickSort(arr, pi + 1, high); // 对右半部分递归排序
}
}
// 测试函数
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++)
cout << arr[i] << " ";
cout << endl;
}
int main() {
int arr[] = {9, 7, 5, 11, 12, 2, 14, 3, 10};
int n = sizeof(arr) / sizeof(arr[0]);
cout << "Original array: \n";
printArray(arr, n);
quickSort(arr, 0, n - 1);
cout << "\nSorted array: \n";
printArray(arr, n);
return 0;
}
```
阅读全文
相关推荐
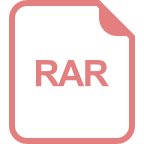
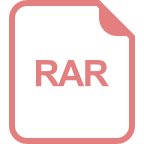
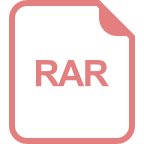
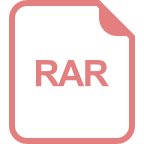
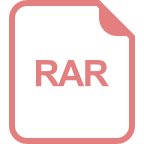
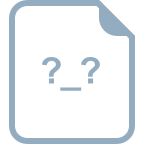
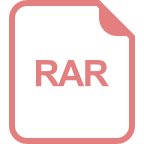
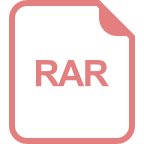
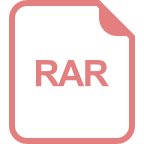
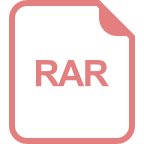
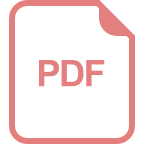
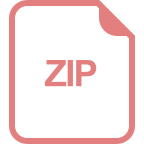