C# XML与list互转
时间: 2024-11-24 20:01:25 浏览: 9
在C#中,XML(可扩展标记语言)可以用于数据持久化和配置文件管理,而List<T>是一种动态数组,常用于存储同类型的元素。如果你想将XML转换为List<T>,或者反过来,可以使用System.Xml.Linq命名空间提供的类如XDocument和 XElement。以下是两个基本示例:
### 将List<T>转换为XML
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml.Linq;
public static void ListToXml(List<MyClass> list, string fileName)
{
var doc = new XDocument(new XElement("Items",
list.Select(item => new XElement("Item",
new XElement("Name", item.Name),
new XElement("Value", item.Value)))));
doc.Save(fileName);
}
// 其中 MyClass 是你想要序列化的自定义类,例如:
public class MyClass
{
public string Name { get; set; }
public int Value { get; set; }
}
```
### 将XML转换为List<T>
```csharp
public static List<MyClass> XmlToList(string xmlFilePath)
{
var doc = XDocument.Load(xmlFilePath);
return doc.Descendants("Item")
.Select(x => new MyClass
{
Name = (string)x.Element("Name"),
Value = (int)x.Element("Value")
})
.ToList();
}
```
在这个例子中,`Descendants()`方法会查找所有名为"Item"的元素,并通过`.Element()`获取每个元素的子元素。
阅读全文
相关推荐
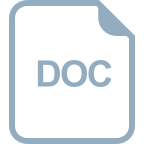
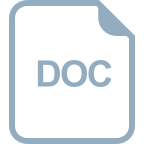
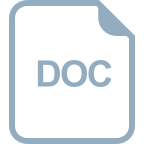
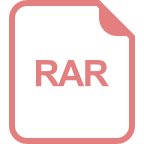
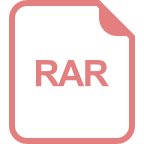
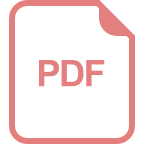
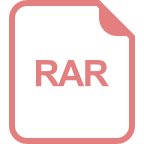
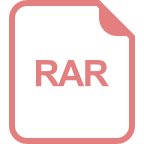
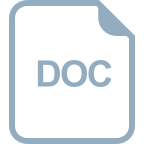
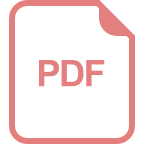
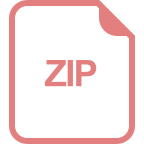
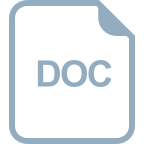
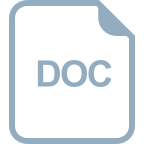
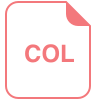
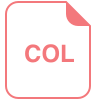


