iview的switch用 1 和 0 来判断是否选中时会很有用
时间: 2024-10-15 08:16:13 浏览: 40
iView是一个基于Vue.js的前端组件库,它提供了一个名为`Switch`的开关按钮组件,用于用户交互中选择或切换选项。当使用`Switch`时,通常通过将`value`属性绑定到一个布尔类型的变量上,比如1代表选中,0代表未选中。这样设计的好处是:
1. 简洁明了:数值1和0直观地对应于开关的状态,方便开发者理解和维护代码逻辑。
2. 数据驱动:状态的变化可以直接通过数据流更新,无需额外的手动控制,提高了代码的灵活性。
3. 可复用性强:如果需要在多个地方显示相同的开关状态,只需要改变数据源的值即可,无需复制粘贴大量相似的HTML和事件处理代码。
例如,你可以这样做:
```html
<template>
<iv-switch v-model="isOn" @on-change="handleToggle"></iv-switch>
</template>
<script>
export default {
data() {
return {
isOn: 0 // 初始状态为未选中
};
},
methods: {
handleToggle(value) {
console.log('开关状态改变', value);
this.isOn = value; // 更新开关状态
}
}
};
</script>
```
相关问题
iview tree 选中
要在 iView Tree 中选中某个节点,可以使用 `selected` 属性来指定选中的节点。例如:
```html
<template>
<div>
<Tree :data="data" :selected="selectedNode" @on-select="onSelect"></Tree>
</div>
</template>
<script>
export default {
data() {
return {
data: [
{
title: "Node 1",
key: "1",
children: [
{
title: "Node 1-1",
key: "1-1"
},
{
title: "Node 1-2",
key: "1-2"
}
]
},
{
title: "Node 2",
key: "2",
children: [
{
title: "Node 2-1",
key: "2-1"
},
{
title: "Node 2-2",
key: "2-2"
}
]
}
],
selectedNode: "1-1"
};
},
methods: {
onSelect(node) {
console.log(node);
}
}
};
</script>
```
在上述代码中,我们指定了 `selectedNode` 属性为 `"1-1"`,表示默认选中树形结构中的 `"Node 1-1"` 节点。同时,我们还定义了 `on-select` 事件来监听节点选中事件,当用户点击节点时,会触发该事件,并将选中的节点信息传递给 `onSelect` 方法。
iview table和switch一起使用时怎么使返回值selection中包含switch的值
在 iView Table 中,当使用 Switch 组件时,我们可以通过监听 Switch 组件的 change 事件,将其选中状态的值加入到 Table 的 selection 中。
以下是示例代码:
```html
<template>
<Table :data="tableData" :columns="columns" :row-selection="rowSelection"></Table>
</template>
<script>
export default {
data () {
return {
tableData: [
{
name: 'John',
age: 23,
married: false
},
{
name: 'Mary',
age: 25,
married: true
},
{
name: 'Tom',
age: 28,
married: false
}
],
columns: [
{
title: 'Name',
key: 'name'
},
{
title: 'Age',
key: 'age'
},
{
title: 'Married',
key: 'married',
render: (h, params) => {
return h('Switch', {
props: {
value: params.row.married
},
on: {
change: (value) => {
params.row.married = value;
this.updateSelection();
}
}
});
}
}
],
rowSelection: {
onChange: (selectedRows) => {
this.selectedRows = selectedRows;
},
selectedRowKeys: []
}
};
},
methods: {
updateSelection () {
this.rowSelection.selectedRowKeys = this.tableData.filter(item => item.married).map(item => item.key);
}
}
};
</script>
```
在上面的示例代码中,我们在 Table 的 columns 中使用了 Switch 组件,并监听了其 change 事件。当 Switch 组件的选中状态发生改变时,我们将其选中状态的值加入到 Table 的 selection 中。具体来说,我们在 change 事件中,更新了当前行数据的 married 属性,并调用了 updateSelection 方法,该方法会根据当前表格数据中 married 为 true 的行,更新 rowSelection 中的 selectedRowKeys 属性。最终,我们可以通过监听 Table 的 onChange 事件,获取到 selection 中的数据。
阅读全文
相关推荐
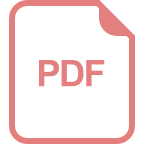
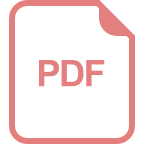
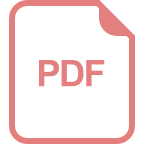

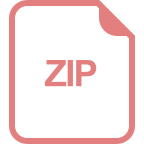
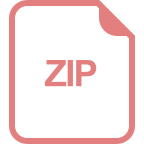
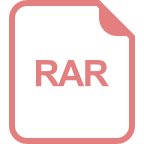









