c# mongodb convert between list and bson
时间: 2024-03-02 08:52:54 浏览: 358
To convert between a list and BSON in C# with MongoDB, you can use the `BsonDocument` class and the `BsonArray` class from the MongoDB C# driver.
To convert a list to BSON:
```
List<string> list = new List<string> { "one", "two", "three" };
BsonArray bsonArray = new BsonArray(list);
BsonDocument bsonDocument = new BsonDocument { { "list", bsonArray } };
```
To convert BSON to a list:
```
BsonDocument bsonDocument = ... // retrieve the document from MongoDB
BsonArray bsonArray = bsonDocument["list"].AsBsonArray;
List<string> list = bsonArray.Select(x => x.AsString).ToList();
```
Note that you should replace the `...` with the code that retrieves the document from MongoDB.
相关问题
c# mongodb convert between list and bson, and save bson in asp.net web core session
To save the BSON in ASP.NET Core session, you can serialize the BSON to a byte array and store it in the session as a string. Here's an example:
```
using MongoDB.Bson;
using MongoDB.Bson.IO;
using MongoDB.Bson.Serialization;
using MongoDB.Driver;
using Microsoft.AspNetCore.Http;
using System.IO;
using System.Text;
// Convert a list to BSON
List<string> list = new List<string> { "one", "two", "three" };
BsonArray bsonArray = new BsonArray(list);
BsonDocument bsonDocument = new BsonDocument { { "list", bsonArray } };
// Serialize the BSON to a byte array
var memoryStream = new MemoryStream();
var writer = new BsonBinaryWriter(memoryStream);
BsonSerializer.Serialize(writer, typeof(BsonDocument), bsonDocument);
byte[] bsonBytes = memoryStream.ToArray();
// Convert the byte array to a string
string bsonString = Encoding.UTF8.GetString(bsonBytes);
// Save the BSON in session
HttpContext.Session.SetString("bson", bsonString);
```
To retrieve the BSON from session and convert it back to a list:
```
// Retrieve the BSON from session
string bsonString = HttpContext.Session.GetString("bson");
byte[] bsonBytes = Encoding.UTF8.GetBytes(bsonString);
// Deserialize the BSON to a BsonDocument
var memoryStream = new MemoryStream(bsonBytes);
var reader = new BsonBinaryReader(memoryStream);
BsonDocument bsonDocument = BsonSerializer.Deserialize<BsonDocument>(reader);
// Convert the BsonDocument to a list
BsonArray bsonArray = bsonDocument["list"].AsBsonArray;
List<string> list = bsonArray.Select(x => x.AsString).ToList();
```
Note that you need to add the `MongoDB.Driver` and `Microsoft.AspNetCore.Session` NuGet packages to your project to use the MongoDB C# driver and ASP.NET Core session.
c# mongodb convert between model and bson
To convert between a model and BSON in C# with MongoDB, you can use the `BsonDocument` class and the `BsonSerializer` class from the MongoDB C# driver.
To convert a model to BSON:
```
using MongoDB.Bson;
using MongoDB.Bson.Serialization;
public class Person
{
public ObjectId Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
}
Person person = new Person { Name = "John", Age = 30 };
BsonDocument bsonDocument = person.ToBsonDocument();
```
To convert BSON to a model:
```
using MongoDB.Bson;
using MongoDB.Bson.Serialization;
BsonDocument bsonDocument = ... // retrieve the document from MongoDB
Person person = BsonSerializer.Deserialize<Person>(bsonDocument);
```
Note that you should replace the `...` with the code that retrieves the document from MongoDB.
If you need to convert a list of models to BSON, you can use `BsonArray` and `ToBsonDocumentArray`:
```
List<Person> people = new List<Person>
{
new Person { Name = "John", Age = 30 },
new Person { Name = "Jane", Age = 25 }
};
BsonArray bsonArray = new BsonArray(people.Select(x => x.ToBsonDocument()));
BsonDocument bsonDocument = new BsonDocument { { "people", bsonArray } };
```
And to convert BSON to a list of models:
```
BsonDocument bsonDocument = ... // retrieve the document from MongoDB
BsonArray bsonArray = bsonDocument["people"].AsBsonArray;
List<Person> people = bsonArray.Select(x => BsonSerializer.Deserialize<Person>(x.AsBsonDocument)).ToList();
```
Again, you should replace the `...` with the code that retrieves the document from MongoDB.
阅读全文
相关推荐
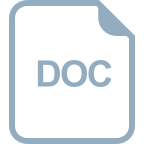
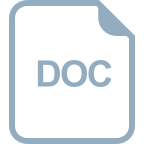
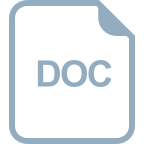

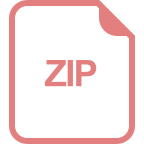
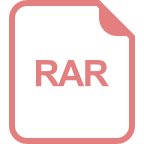
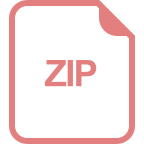
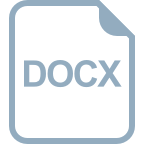
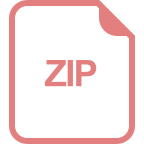
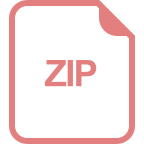
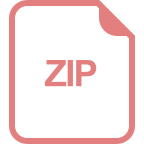
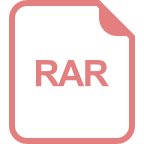
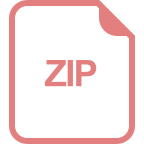
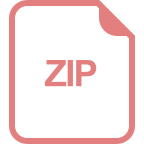
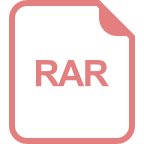
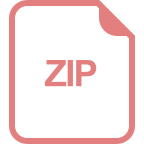
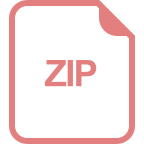