ubuntu中springboot实现word转pdf
时间: 2024-02-28 08:54:19 浏览: 138
在Ubuntu中实现Word转PDF,您可以按照以下步骤进行操作:
1. 安装OpenJDK和Maven:
```
sudo apt-get update
sudo apt-get install openjdk-8-jdk
sudo apt-get install maven
```
2. 创建一个Spring Boot项目:
```
mvn archetype:generate -DgroupId=com.example -DartifactId=word2pdf -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
```
3. 在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
```
4. 创建一个Controller类,其中包含一个方法,该方法接受一个Word文件并将其转换为PDF文件:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import com.itextpdf.text.Document;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfWriter;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
@Controller
public class WordToPdfController {
@PostMapping("/convert")
public String convertToPdf(@RequestParam("file") MultipartFile file) {
try {
// Read the Word document
XWPFDocument docx = new XWPFDocument(new FileInputStream(convert(file)));
// Create the PDF document
Document pdf = new Document();
PdfWriter.getInstance(pdf, new FileOutputStream("converted.pdf"));
pdf.open();
// Read each paragraph from the Word document and write it to the PDF document
for (XWPFParagraph para : docx.getParagraphs()) {
for (XWPFRun run : para.getRuns()) {
// Add the text to the PDF document
pdf.add(new Paragraph(run.getText(0)));
}
}
pdf.close();
return "success";
} catch (Exception e) {
e.printStackTrace();
return "error";
}
}
// Convert MultipartFile to File
private File convert(MultipartFile file) throws IOException {
File convFile = new File(file.getOriginalFilename());
convFile.createNewFile();
FileOutputStream fos = new FileOutputStream(convFile);
fos.write(file.getBytes());
fos.close();
return convFile;
}
}
```
此代码将从客户端接收一个MultipartFile对象,将其转换为File对象,然后使用Apache POI读取Word文档并使用iText将其写入PDF文件。
5. 启动应用程序并使用Postman或浏览器发送请求,将Word文件作为MultipartFile对象发送到“/convert”端点。在应用程序目录中,您将找到一个名为“converted.pdf”的新文件,其中包含转换后的PDF文档。
```
mvn spring-boot:run
```
阅读全文
相关推荐
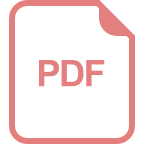
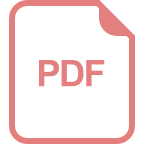
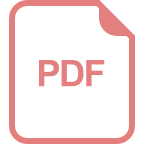
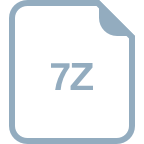
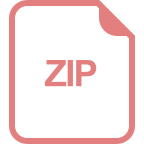
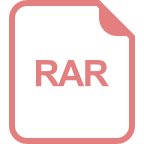
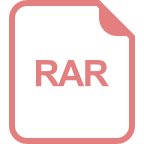
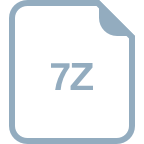
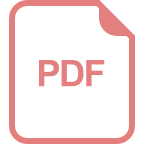
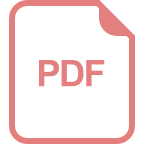
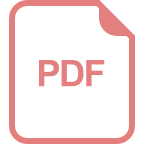
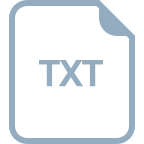
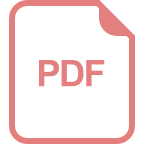
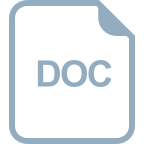