c语言实现 1 使用结构体数据结构 2 随机抽取一个单词,界面显示该单词的首尾字母以及中间空缺位符,对应的释义,缺失的部分由玩家输入,显示游戏剩余的错误次数,显示出题目的同时开始计时 3 玩家输入完成后,判断其是否正确,当答题剩余3秒时进行提示
时间: 2023-11-18 21:02:58 浏览: 200
以下是一个基于C语言的实现,包含结构体数据结构和游戏逻辑实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
// 定义单词结构体
struct Word {
char word[20]; // 单词
char meaning[50]; // 释义
};
// 定义游戏状态结构体
struct GameState {
struct Word word; // 当前单词
char hidden[20]; // 隐藏的单词
int errors; // 剩余错误次数
time_t start_time; // 开始时间
};
// 单词数组
struct Word words[] = {
{"apple", "a fruit"},
{"banana", "a fruit"},
{"computer", "an electronic device"},
{"language", "a system of communication"},
{"program", "a set of instructions"},
{"keyboard", "an input device"},
{"mouse", "an input device"},
{"printer", "an output device"},
{"monitor", "an output device"},
{"algorithm", "a step-by-step procedure for solving a problem"},
{"database", "a collection of data"},
{"network", "a group of interconnected devices"},
{"server", "a computer or program that manages access to a centralized resource"},
{"client", "a computer or program that requests services from a server"},
};
int word_count = sizeof(words) / sizeof(words[0]); // 单词数量
// 随机选择一个单词
struct Word select_word() {
int index = rand() % word_count;
return words[index];
}
// 初始化游戏状态
struct GameState init_game() {
struct GameState state;
state.word = select_word();
int word_len = strlen(state.word.word);
for (int i = 0; i < word_len; i++) {
state.hidden[i] = '_';
}
state.hidden[word_len] = '\0';
state.errors = 5;
state.start_time = time(NULL);
return state;
}
// 显示游戏状态
void display_game(struct GameState state) {
printf("Word: %c%s%c\n", state.word.word[0], state.hidden + 1, state.word.word[strlen(state.word.word) - 1]);
printf("Meaning: %s\n", state.word.meaning);
printf("Errors left: %d\n", state.errors);
}
// 判断玩家输入是否正确
int check_guess(struct GameState *state, char guess) {
int correct = 0;
int word_len = strlen(state->word.word);
for (int i = 0; i < word_len; i++) {
if (state->word.word[i] == guess) {
state->hidden[i] = guess;
correct = 1;
}
}
if (!correct) {
state->errors--;
}
if (strcmp(state->hidden, state->word.word) == 0) {
return 1;
}
return 0;
}
// 主函数
int main() {
srand(time(NULL));
struct GameState state = init_game();
display_game(state);
while (1) {
printf("Guess a letter: ");
char guess;
scanf("%c", &guess);
if (check_guess(&state, guess)) {
printf("You win!\n");
break;
}
if (state.errors == 0) {
printf("You lose!\n");
break;
}
display_game(state);
time_t elapsed_time = time(NULL) - state.start_time;
if (elapsed_time >= 7) {
printf("Hint: The word starts with '%c' and ends with '%c'\n", state.word.word[0], state.word.word[strlen(state.word.word) - 1]);
}
getchar(); // 消耗回车符
}
return 0;
}
```
在每次玩家猜测后,我们通过 `check_guess` 函数来检查玩家猜测的字母是否正确,如果正确则更新隐藏单词状态,并判断是否已猜测完整个单词;如果错误则减少错误次数。当剩余错误次数为0时,游戏结束并宣布失败;当猜测到整个单词时,游戏结束并宣布胜利。在每次猜测后,我们通过 `display_game` 函数来显示当前游戏状态。此外,我们还添加了一个计时器来在猜测过程中提供提示,当猜测时间超过7秒时,程序会自动给出一个提示,显示单词的首尾字母。
可以根据需要对本示例进行修改和扩展。
阅读全文
相关推荐
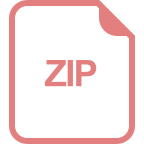
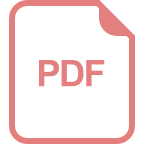
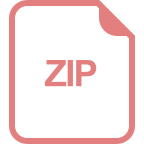
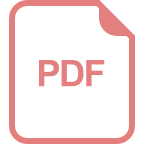
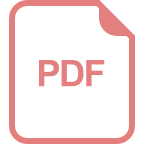
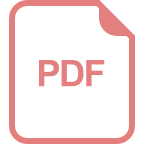
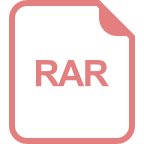
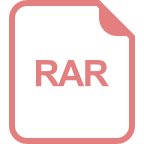
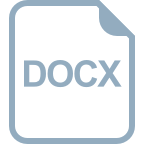
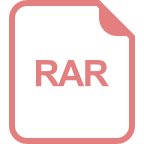
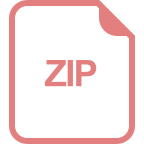
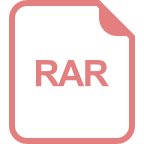
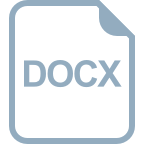