c#取指定句柄的内容信息
时间: 2024-03-27 17:36:19 浏览: 67
你可以使用 Windows API 来实现获取指定句柄的内容信息。具体实现步骤如下:
1. 引入 Windows API 相关命名空间:
```
using System.Runtime.InteropServices;
using System.Text;
```
2. 定义 Windows API 函数:
```
[DllImport("user32.dll", SetLastError = true)]
static extern int GetWindowText(IntPtr hWnd, StringBuilder lpString, int nMaxCount);
[DllImport("user32.dll", SetLastError = true)]
static extern int GetWindowTextLength(IntPtr hWnd);
[DllImport("user32.dll", SetLastError = true)]
static extern int GetClassName(IntPtr hWnd, StringBuilder lpClassName, int nMaxCount);
[DllImport("user32.dll", SetLastError = true)]
static extern int SendMessage(IntPtr hWnd, uint Msg, int wParam, StringBuilder lParam);
```
3. 实现获取指定句柄的内容信息的方法:
```
public static string GetWindowText(IntPtr hwnd)
{
int length = GetWindowTextLength(hwnd);
StringBuilder sb = new StringBuilder(length + 1);
GetWindowText(hwnd, sb, sb.Capacity);
return sb.ToString();
}
public static string GetClassName(IntPtr hwnd)
{
StringBuilder sb = new StringBuilder(256);
GetClassName(hwnd, sb, sb.Capacity);
return sb.ToString();
}
public static string GetControlText(IntPtr hwnd)
{
const uint WM_GETTEXTLENGTH = 0x000E;
const uint WM_GETTEXT = 0x000D;
int length = (int)SendMessage(hwnd, WM_GETTEXTLENGTH, 0, null);
StringBuilder sb = new StringBuilder(length + 1);
SendMessage(hwnd, WM_GETTEXT, sb.Capacity, sb);
return sb.ToString();
}
```
其中,`GetWindowText()` 函数用于获取窗口标题,`GetClassName()` 函数用于获取窗口类名,`GetControlText()` 函数用于获取控件文本内容,`SendMessage()` 函数用于向窗口发送消息。
使用示例:
```
IntPtr hwnd = new IntPtr(0x12345678); // 替换为你要获取的句柄值
string windowTitle = GetWindowText(hwnd);
string windowClass = GetClassName(hwnd);
string controlText = GetControlText(hwnd);
```
阅读全文
相关推荐
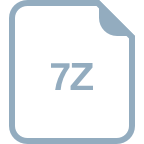
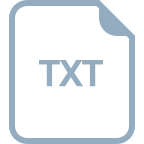
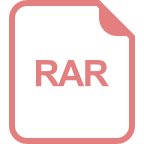
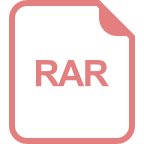
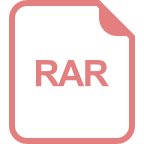
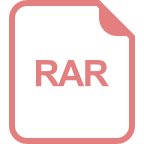
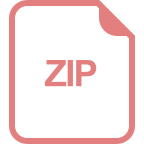
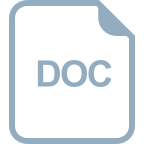
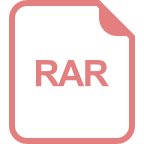
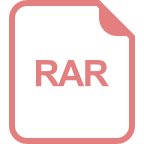
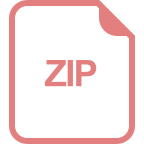
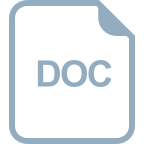
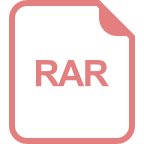
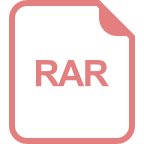
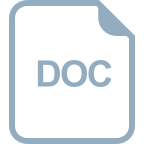
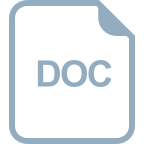