安卓开发:设计一个照片墙,可单击“上一页”、“下一页”按钮进行翻页
时间: 2023-10-01 14:07:37 浏览: 78
要设计一个照片墙,你可以使用GridView来展示图片。在GridView中,你可以设置每个单元格的大小,以及每行显示的列数。然后,你可以使用适配器来填充GridView中的数据。在适配器中,你可以加载图片,并将其显示在每个单元格中。
为了实现翻页功能,你可以在页面上添加两个按钮:上一页和下一页。当用户点击这些按钮时,你可以更新适配器中的数据,以显示下一页或上一页的图片。例如,你可以维护一个当前页码的变量,并在点击按钮时更新该变量。然后,你可以计算要显示的图片的起始位置,并更新适配器中的数据。
下面是一个实现照片墙和翻页功能的示例代码:
```java
public class PhotoWallActivity extends AppCompatActivity {
private static final int PAGE_SIZE = 16;
private GridView mPhotoWall;
private Button mPrevButton;
private Button mNextButton;
private int mCurrentPage = 0;
private List<String> mPhotos = new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_photo_wall);
mPhotoWall = findViewById(R.id.photo_wall);
mPrevButton = findViewById(R.id.prev_button);
mNextButton = findViewById(R.id.next_button);
// Load all photos into mPhotos
for (int i = 0; i < 100; i++) {
mPhotos.add("http://example.com/photo/" + i + ".jpg");
}
mPhotoWall.setNumColumns(4); // Display 4 columns per row
mPhotoWall.setAdapter(new PhotoAdapter(getPagePhotos())); // Display the first page
mPrevButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (mCurrentPage > 0) {
mCurrentPage--;
mPhotoWall.setAdapter(new PhotoAdapter(getPagePhotos()));
}
}
});
mNextButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (mCurrentPage < getTotalPages() - 1) {
mCurrentPage++;
mPhotoWall.setAdapter(new PhotoAdapter(getPagePhotos()));
}
}
});
}
private List<String> getPagePhotos() {
int start = mCurrentPage * PAGE_SIZE;
int end = Math.min(start + PAGE_SIZE, mPhotos.size());
return mPhotos.subList(start, end);
}
private int getTotalPages() {
return (int) Math.ceil((double) mPhotos.size() / PAGE_SIZE);
}
private class PhotoAdapter extends BaseAdapter {
private List<String> mPhotos;
public PhotoAdapter(List<String> photos) {
mPhotos = photos;
}
@Override
public int getCount() {
return mPhotos.size();
}
@Override
public Object getItem(int position) {
return mPhotos.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ImageView imageView;
if (convertView == null) {
imageView = new ImageView(PhotoWallActivity.this);
imageView.setLayoutParams(new GridView.LayoutParams(
ViewGroup.LayoutParams.MATCH_PARENT, 300));
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
} else {
imageView = (ImageView) convertView;
}
// Load the photo at the specified position
Glide.with(PhotoWallActivity.this).load(mPhotos.get(position)).into(imageView);
return imageView;
}
}
}
```
在这个示例代码中,我们使用GridView来展示图片,并使用Glide库加载图片。我们使用一个PhotoAdapter来填充GridView中的数据,其中getPagePhotos()方法返回当前页的图片列表。我们还使用mCurrentPage变量来记录当前页码,并在点击上一页和下一页按钮时更新它。在每次更新页码后,我们使用新的PhotoAdapter来更新GridView中的数据。
阅读全文
相关推荐
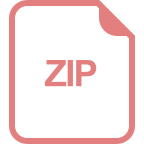
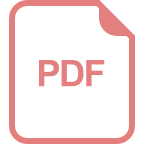
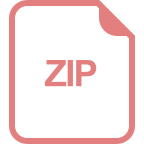















