利matlab遗传算法解决AGV和L-AGV的调度及路径优化问题并写出代码
时间: 2023-09-12 13:11:58 浏览: 125
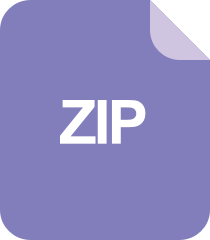
用遗传算法解决车辆优化调度问题

当然可以,以下是一个基于Matlab的遗传算法解决AGV和L-AGV的调度及路径优化问题的示例代码。在代码中,我们假设有两个AGV和两个任务需要完成。
```matlab
% 定义任务和位置
tasks = [1 2];
positions = [1 2 3 4];
% 定义染色体和适应度函数
num_genes = 4;
chromosome_length = num_genes * 2;
fitness_func = @(chromosome) fitness(chromosome, tasks, positions);
% 初始化种群
population_size = 50;
population = zeros(population_size, chromosome_length);
for i = 1:population_size
genes = zeros(1, chromosome_length);
for j = 1:chromosome_length
if mod(j, 2) == 1
% 选择任务
genes(j) = tasks(randi(length(tasks)));
else
% 选择位置
genes(j) = positions(randi(length(positions)));
end
end
population(i, :) = genes;
end
% 遗传算法参数
num_generations = 100;
mutation_rate = 0.01;
% 迭代
for generation = 1:num_generations
% 计算适应度
fitness_values = zeros(1, population_size);
for i = 1:population_size
fitness_values(i) = fitness_func(population(i, :));
end
% 排序
[sorted_fitness_values, indices] = sort(fitness_values);
sorted_population = population(indices, :);
% 筛选
elite_size = 5;
elite = sorted_population(1:elite_size, :);
selection_size = population_size - elite_size;
selected = roulette_wheel_selection(sorted_population, sorted_fitness_values, selection_size);
% 交叉
offspring = zeros(selection_size, chromosome_length);
for i = 1:2:selection_size
parent1 = selected(i, :);
parent2 = selected(i+1, :);
[child1, child2] = single_point_crossover(parent1, parent2);
offspring(i, :) = child1;
offspring(i+1, :) = child2;
end
% 变异
for i = 1:selection_size
if rand() < mutation_rate
offspring(i, :) = mutation(offspring(i, :), tasks, positions);
end
end
% 合并父代和子代
population = [elite; offspring];
end
% 返回最优解
best_chromosome = elite(1, :);
best_fitness = fitness_func(best_chromosome);
disp(['最优解: ', num2str(best_chromosome)]);
disp(['最优解适应度: ', num2str(best_fitness)]);
% 定义适应度函数
function fitness_value = fitness(chromosome, tasks, positions)
num_genes = length(chromosome) / 2;
task1 = 0;
task2 = 0;
for i = 1:num_genes
task = chromosome(2*i-1);
pos = chromosome(2*i);
if task == 1
task1 = pos;
else
task2 = pos;
end
end
dist1 = abs(task1 - 1) + abs(task2 - 3);
dist2 = abs(task1 - 2) + abs(task2 - 4);
fitness_value = - (dist1 + dist2);
end
% 轮盘赌选择
function selected = roulette_wheel_selection(population, fitness_values, selection_size)
total_fitness = sum(fitness_values);
probabilities = fitness_values / total_fitness;
cumulative_probabilities = cumsum(probabilities);
selected = zeros(selection_size, size(population, 2));
for i = 1:selection_size
r = rand();
j = find(cumulative_probabilities >= r, 1, 'first');
selected(i, :) = population(j, :);
end
end
% 单点交叉
function [child1, child2] = single_point_crossover(parent1, parent2)
chromosome_length = length(parent1);
crossover_point = randi(chromosome_length-1);
child1 = [parent1(1:crossover_point) parent2(crossover_point+1:end)];
child2 = [parent2(1:crossover_point) parent1(crossover_point+1:end)];
end
% 变异
function mutated_chromosome = mutation(chromosome, tasks, positions)
chromosome_length = length(chromosome);
gene_index = randi(chromosome_length);
if mod(gene_index, 2) == 1
% 变异任务
chromosome(gene_index) = tasks(randi(length(tasks)));
else
% 变异位置
chromosome(gene_index) = positions(randi(length(positions)));
end
mutated_chromosome = chromosome;
end
```
在这个示例代码中,我们使用了单点交叉和随机变异两种操作,并使用轮盘赌选择策略来选择父代染色体。最终,我们返回了最优解及其适应度。需要注意的是,这个示例代码只是一个简单的示例,实际应用中需要根据具体问题进行调整和优化。
阅读全文
相关推荐
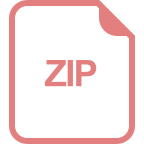
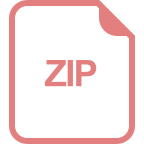
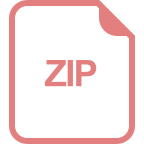
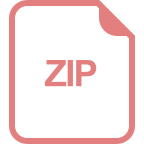
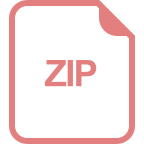
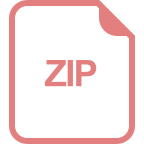
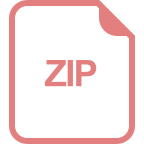
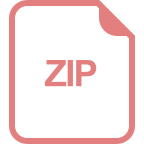
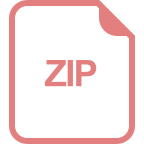
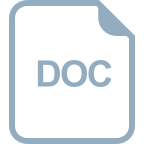
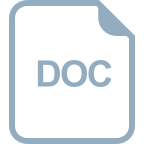
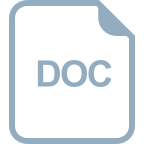
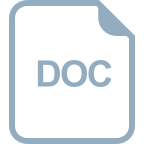
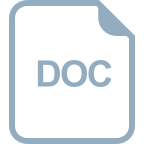


