在 QT 中使用 TiDB 数据库
时间: 2023-11-10 07:06:36 浏览: 177
要在QT中使用TiDB数据库,你需要完成以下步骤:
1. 下载并安装MySQL Connector/C++(TiDB是兼容MySQL协议的)。
2. 在QT项目中包含MySQL Connector/C++的头文件。
3. 使用MySQL Connector/C++提供的API连接到TiDB数据库。
4. 执行SQL语句来操作TiDB数据库。
下面是一个简单的示例代码,它连接到TiDB数据库并查询一个表:
```cpp
#include <QCoreApplication>
#include <iostream>
#include <mysql_connection.h>
#include <mysql_driver.h>
#include <cppconn/exception.h>
#include <cppconn/resultset.h>
#include <cppconn/statement.h>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
try {
// 创建一个MySQL连接
sql::mysql::MySQL_Driver *driver;
sql::Connection *con;
driver = sql::mysql::get_mysql_driver_instance();
con = driver->connect("tcp://localhost:4000", "root", "password");
// 设置数据库编码
con->setSchema("mydatabase");
con->setClientOption("characterSetResults", "utf8");
// 执行SQL语句
sql::Statement *stmt;
sql::ResultSet *res;
stmt = con->createStatement();
res = stmt->executeQuery("SELECT * FROM mytable");
// 处理查询结果
while (res->next()) {
std::cout << res->getInt("id") << ", ";
std::cout << res->getString("name") << std::endl;
}
// 释放资源
delete res;
delete stmt;
delete con;
} catch (sql::SQLException &e) {
std::cout << "# ERR: SQLException in " << __FILE__;
std::cout << "(" << __FUNCTION__ << ") on line " << __LINE__ << std::endl;
std::cout << "# ERR: " << e.what();
std::cout << " (MySQL error code: " << e.getErrorCode();
std::cout << ", SQLState: " << e.getSQLState() << " )" << std::endl;
}
return a.exec();
}
```
注意,TiDB使用MySQL协议,但是与传统MySQL的一些特性有所不同,例如,TiDB是分布式的、支持事务的数据库,因此你需要根据TiDB的文档来正确地使用它。
阅读全文
相关推荐
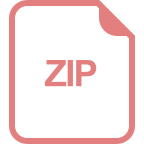
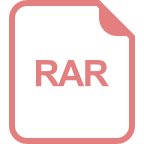
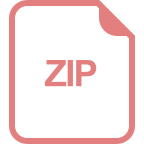
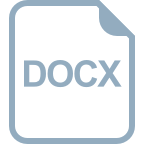
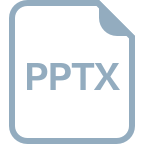
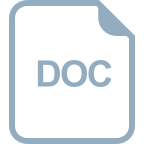
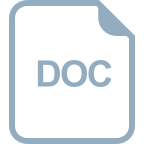
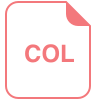







