C# 去掉外部程序的窗体激活状态监听
时间: 2023-07-12 12:15:11 浏览: 137
要去除外部程序的窗体激活状态监听,可以使用`SetWindowLong`函数来修改窗口样式。具体步骤如下:
1. 首先,需要引入Win32 API函数:
```csharp
[DllImport("user32.dll")]
public static extern int SetWindowLong(IntPtr hWnd, int nIndex, int dwNewLong);
```
2. 然后,在启动外部程序前,需要获取该程序的窗口句柄:
```csharp
Process process = new Process();
process.StartInfo.FileName = "path/to/your/program.exe";
process.Start();
process.WaitForInputIdle();
IntPtr hWnd = process.MainWindowHandle;
```
这里使用`process.MainWindowHandle`获取外部程序的主窗口句柄。
3. 最后,使用`SetWindowLong`函数来修改窗口样式:
```csharp
const int GWL_EXSTYLE = -20;
const int WS_EX_NOACTIVATE = 0x08000000;
int style = GetWindowLong(hWnd, GWL_EXSTYLE);
SetWindowLong(hWnd, GWL_EXSTYLE, style | WS_EX_NOACTIVATE);
```
在这个示例中,我们使用`SetWindowLong`函数将窗口样式修改为`WS_EX_NOACTIVATE`,这样程序窗口就不会被激活。
完整代码如下:
```csharp
using System.Diagnostics;
using System.Runtime.InteropServices;
[DllImport("user32.dll")]
public static extern int SetWindowLong(IntPtr hWnd, int nIndex, int dwNewLong);
[DllImport("user32.dll")]
public static extern int GetWindowLong(IntPtr hWnd, int nIndex);
const int GWL_EXSTYLE = -20;
const int WS_EX_NOACTIVATE = 0x08000000;
Process process = new Process();
process.StartInfo.FileName = "path/to/your/program.exe";
process.Start();
process.WaitForInputIdle();
IntPtr hWnd = process.MainWindowHandle;
int style = GetWindowLong(hWnd, GWL_EXSTYLE);
SetWindowLong(hWnd, GWL_EXSTYLE, style | WS_EX_NOACTIVATE);
```
在这个示例中,我们使用了`GetWindowLong`函数来获取窗口原有的样式,然后使用`SetWindowLong`函数将其修改为`WS_EX_NOACTIVATE`。
阅读全文
相关推荐
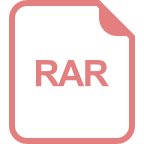
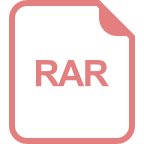
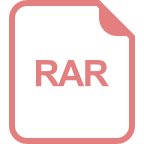
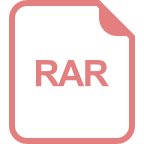
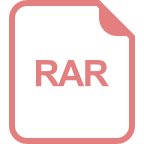


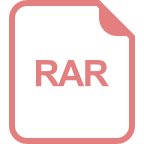
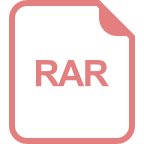
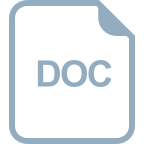

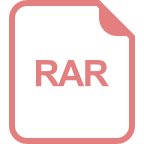


