vue框架element ui 导航菜单路由
时间: 2023-06-24 10:02:59 浏览: 134
在 Vue 框架中,我们可以使用 Element UI 组件库中的 `el-menu` 和 `el-menu-item` 组件来实现导航菜单。同时,我们可以使用 Vue Router 来实现路由跳转。
以下是一个基本的例子:
```html
<template>
<div>
<el-menu default-active="1" class="el-menu-vertical-demo" @select="handleSelect">
<el-menu-item index="1">
<router-link to="/home">首页</router-link>
</el-menu-item>
<el-menu-item index="2">
<router-link to="/about">关于我们</router-link>
</el-menu-item>
<el-menu-item index="3">
<router-link to="/contact">联系我们</router-link>
</el-menu-item>
</el-menu>
<router-view></router-view>
</div>
</template>
<script>
export default {
methods: {
handleSelect(index) {
console.log(index);
},
},
};
</script>
```
在上面的例子中,我们先使用 `el-menu` 组件创建了一个垂直方向的菜单,并设置了默认选中的菜单项为第一个。然后,我们使用 `el-menu-item` 组件创建了三个菜单项,并在每个菜单项中使用 `router-link` 组件来设置路由跳转的目标路径。
最后,我们使用 `router-view` 组件来渲染实际的页面内容。
在 Vue Router 中,我们需要在路由配置中设置每个路由对应的组件,例如:
```javascript
import Vue from "vue";
import VueRouter from "vue-router";
import Home from "@/views/Home.vue";
import About from "@/views/About.vue";
import Contact from "@/views/Contact.vue";
Vue.use(VueRouter);
const routes = [
{
path: "/",
redirect: "/home",
},
{
path: "/home",
component: Home,
},
{
path: "/about",
component: About,
},
{
path: "/contact",
component: Contact,
},
];
const router = new VueRouter({
mode: "history",
base: process.env.BASE_URL,
routes,
});
export default router;
```
在路由配置中,我们首先设置了根路径 `/` 的重定向到 `/home`,然后分别设置了 `/home`、`/about` 和 `/contact` 路径对应的组件。
在实际开发中,我们可以根据需要进一步定制菜单的样式和行为,例如添加二级菜单、设置菜单的图标等。
阅读全文
相关推荐
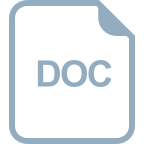
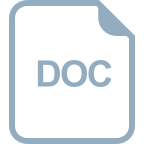
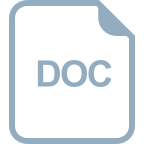
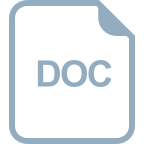
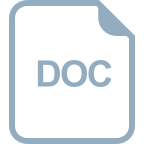
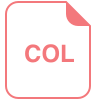
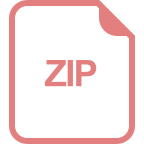
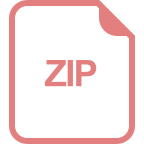
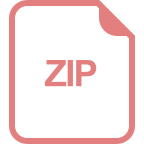
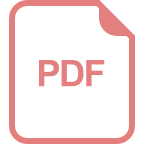
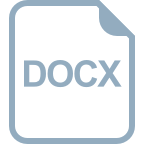
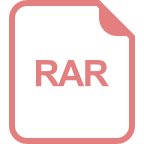
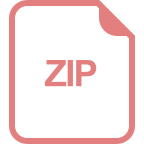
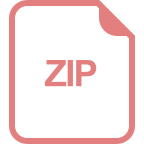
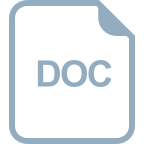
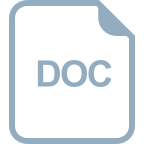

