一个单词表存放了五个表示颜色的英文单词,输入一个字母,在单词表中查找并输出所有以此字母开头的单词,若没有找到,输出Not Found。要求用指针数组和二级指针实现。
时间: 2023-06-12 17:04:40 浏览: 180
使用指针数组实现:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char colors[5][10] = {"red", "green", "blue", "yellow", "black"};
char letter;
cout << "Enter a letter: ";
cin >> letter;
bool found = false;
for (int i = 0; i < 5; i++) {
if (colors[i][0] == letter) {
found = true;
cout << colors[i] << endl;
}
}
if (!found) {
cout << "Not Found" << endl;
}
return 0;
}
```
使用二级指针实现:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char colors[5][10] = {"red", "green", "blue", "yellow", "black"};
char letter;
cout << "Enter a letter: ";
cin >> letter;
bool found = false;
char **ptr = colors;
for (int i = 0; i < 5; i++) {
if (*ptr[0] == letter) {
found = true;
cout << *ptr << endl;
}
ptr++;
}
if (!found) {
cout << "Not Found" << endl;
}
return 0;
}
```
阅读全文
相关推荐
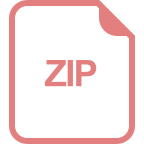
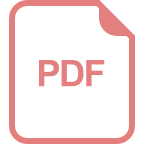



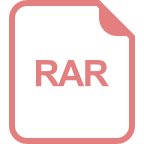
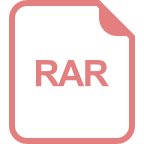
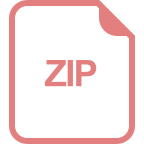
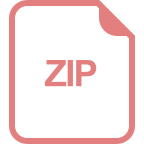
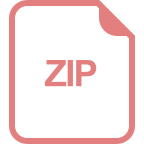