编写一个java类成语接龙的可视化
时间: 2024-03-04 11:51:11 浏览: 15
好的,我可以给您提供一个简单的Java类来实现成语接龙的可视化。这个类使用JavaFX库创建了一个简单的成语接龙游戏界面,并且可以随机生成成语以供接龙。
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Random;
public class ChengYuJieLong extends Application {
private List<String> chengyuList = Arrays.asList(
"千军万马", "一举两得", "四面楚歌", "五湖四海", "七上八下", "八仙过海", "九牛一毛", "十全十美"
);
private List<String> usedChengyuList = new ArrayList<>();
private TextField inputField;
private Label resultLabel;
private Random random = new Random();
@Override
public void start(Stage primaryStage) throws Exception {
BorderPane root = new BorderPane();
// 创建顶部的随机成语按钮和结果显示标签
Button randomButton = new Button("随机成语");
Label randomLabel = new Label();
randomButton.setOnAction(event -> {
String randomChengyu = getRandomChengyu();
randomLabel.setText(randomChengyu);
usedChengyuList.clear();
usedChengyuList.add(randomChengyu);
});
HBox topBox = new HBox(randomButton, randomLabel);
topBox.setSpacing(10);
// 创建中间的输入框和结果标签
inputField = new TextField();
Button submitButton = new Button("提交");
resultLabel = new Label();
submitButton.setOnAction(event -> {
String inputChengyu = inputField.getText();
String lastChengyu = usedChengyuList.get(usedChengyuList.size() - 1);
if (inputChengyu.startsWith(lastChengyu.substring(lastChengyu.length() - 1))) {
resultLabel.setText("接龙成功!");
usedChengyuList.add(inputChengyu);
} else {
resultLabel.setText("接龙失败!");
}
inputField.setText("");
});
HBox middleBox = new HBox(inputField, submitButton);
middleBox.setSpacing(10);
// 创建底部的已用成语列表
VBox bottomBox = new VBox();
Label usedLabel = new Label("已用成语:");
bottomBox.getChildren().add(usedLabel);
// 将界面元素添加到根面板中
root.setTop(topBox);
root.setCenter(middleBox);
root.setBottom(bottomBox);
// 创建场景并将根面板添加到场景中
Scene scene = new Scene(root, 400, 300);
// 设置主舞台的标题和场景
primaryStage.setTitle("成语接龙");
primaryStage.setScene(scene);
// 显示主舞台
primaryStage.show();
}
private String getRandomChengyu() {
return chengyuList.get(random.nextInt(chengyuList.size()));
}
}
```
该类中使用了一个包含几个成语的列表,当用户点击“随机成语”按钮时,随机从列表中选择一个成语,并将其显示在标签上。当用户输入成语并点击“提交”按钮时,程序将检查该成语是否以上一个使用的成语的最后一个字作为开头,如果符合条件,则将其添加到已用成语列表中,并将结果标签设置为“接龙成功”;否则,将结果标签设置为“接龙失败”。
您可以将这个类保存为“ChengYuJieLong.java”,并在命令行中运行它,或者在您的Java IDE中打开并运行它。希望这个类对您有所帮助!
相关推荐
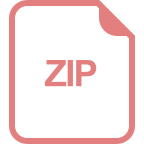
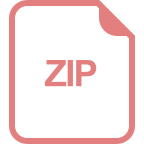














