使用c++编程,从键盘依次输入3位学生的姓名、平时成绩、期中成绩和期末成绩(成绩均为整数),输出总评成绩最高的学生姓名。
时间: 2023-09-07 07:12:38 浏览: 161
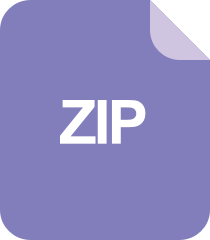
C++期末大作业-基于EasyX和C++语言的可视化学生成绩管理系统源码
好的,让我来回答这个问题。
首先,你可以定义一个结构体来存储学生的信息,包括姓名、平时成绩、期中成绩和期末成绩。然后使用一个循环,从键盘输入每个学生的信息,并将其存储在一个数组中。
接着,再使用另一个循环,遍历数组中的每个学生,计算每个学生的总评成绩并将其存储在一个相应的数组中。
最后,在该数组中找到总评成绩最高的学生,并输出其姓名即可。
以下是一个可能的解决方案的代码示例:
```c
#include <stdio.h>
#define N 3
typedef struct {
char name[20];
int score1;
int score2;
int score3;
} Student;
int main() {
Student students[N];
int total_scores[N];
int max_score = 0;
int max_index = 0;
// 输入学生信息
for (int i = 0; i < N; i++) {
printf("请输入第%d位学生的姓名、平时成绩、期中成绩和期末成绩:", i+1);
scanf("%s%d%d%d", students[i].name, &students[i].score1, &students[i].score2, &students[i].score3);
}
// 计算总评成绩
for (int i = 0; i < N; i++) {
total_scores[i] = students[i].score1 + students[i].score2 + students[i].score3;
}
// 找到总评成绩最高的学生
for (int i = 0; i < N; i++) {
if (total_scores[i] > max_score) {
max_score = total_scores[i];
max_index = i;
}
}
// 输出结果
printf("总评成绩最高的学生是%s\n", students[max_index].name);
return 0;
}
```
希望这个答案对你有所帮助!
阅读全文
相关推荐
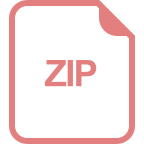















