Run the Newton-Raphson program with different initial points in [-6, 6].
时间: 2024-10-07 09:01:09 浏览: 37
The Newton-Raphson method is an iterative numerical algorithm used to find roots (where a function equals zero) of a real-valued function. It's particularly efficient for finding the zeros of a function that is continuously differentiable.
To run the Newton-Raphson program with different initial points within the interval [-6, 6], you would first need to define your function and its derivative. Let's say you have a function `f(x)` which you want to find the root of, and its derivative `f'(x)`.
Here's a general Python code template using the Newton-Raphson method:
```python
def newton_raphson(f, f_prime, x0, tolerance=1e-6, max_iterations=100):
# Function to be solved
def newton_step(current_x):
return current_x - f(current_x) / f_prime(current_x)
# Check if the initial point is within the given interval
if not (-6 <= x0 <= 6):
print("Initial point should be within [-6, 6].")
return
# Perform iterations
x = x0
for _ in range(max_iterations):
next_x = newton_step(x)
if abs(next_x - x) < tolerance:
break
x = next_x
if abs(f(x)) < tolerance:
return x, "Converged"
else:
return x, "Did not converge"
# Example usage with a function like f(x) = x^2 -6, 7)] # Generate list of points from -6 to 6
for x0 in initial_points:
result, status = newton_raphson(function, derivative, x0)
print(f"Starting from {x0}, Newton-Raphson found a root at {result} with convergence status: {status}")
```
This script will iterate through the specified initial points, apply the Newton-Raphson method, and check for convergence or divergence based on the provided criteria. Make sure to replace `f` and `f_prime` functions with your actual function and its derivative.
阅读全文
相关推荐
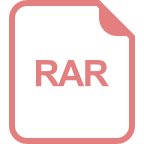
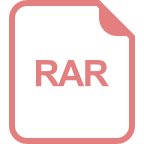
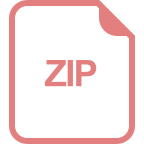
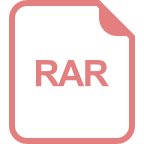
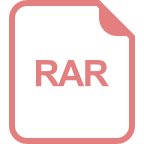
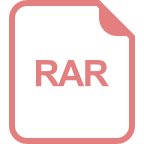
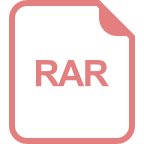
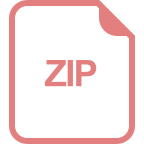
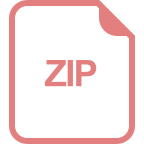
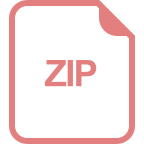
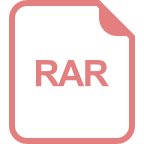
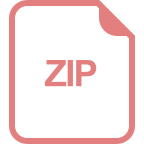
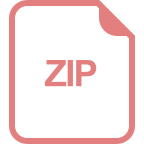
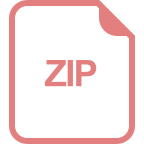
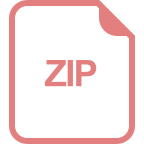
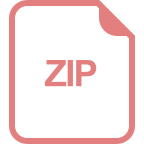
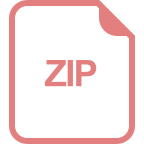
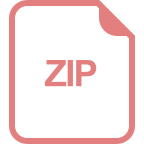