android实现使用AlertDialog.Builder创建对话框加载Layout布局,添加两个TextView水平居中,以及四个RadioButton,并且给四个RadioButton添加点击事件,Dialog有取消以及确定按钮完整布局以及完整功能实现代码
时间: 2024-03-09 22:50:49 浏览: 52
好的,这是一个实现你要求的示例代码:
```
// 定义一个全局变量,用于记录用户选择的RadioButton
int selectedRadioButton = -1;
// 创建AlertDialog.Builder对象
AlertDialog.Builder builder = new AlertDialog.Builder(context);
// 加载自定义布局
View dialogView = LayoutInflater.from(context).inflate(R.layout.dialog_layout, null);
// 获取布局中的控件
TextView textView1 = dialogView.findViewById(R.id.textView1);
TextView textView2 = dialogView.findViewById(R.id.textView2);
RadioButton radioButton1 = dialogView.findViewById(R.id.radioButton1);
RadioButton radioButton2 = dialogView.findViewById(R.id.radioButton2);
RadioButton radioButton3 = dialogView.findViewById(R.id.radioButton3);
RadioButton radioButton4 = dialogView.findViewById(R.id.radioButton4);
// 添加点击事件
radioButton1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
selectedRadioButton = 1;
}
});
radioButton2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
selectedRadioButton = 2;
}
});
radioButton3.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
selectedRadioButton = 3;
}
});
radioButton4.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
selectedRadioButton = 4;
}
});
// 将控件添加到布局中
LinearLayout linearLayout = new LinearLayout(context);
linearLayout.setOrientation(LinearLayout.VERTICAL);
linearLayout.addView(textView1);
linearLayout.addView(textView2);
linearLayout.addView(radioButton1);
linearLayout.addView(radioButton2);
linearLayout.addView(radioButton3);
linearLayout.addView(radioButton4);
linearLayout.setGravity(Gravity.CENTER);
// 将布局添加到Dialog
builder.setView(linearLayout);
// 添加确定按钮
builder.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// 处理用户点击确定按钮的事件
if (selectedRadioButton == -1) {
// 如果用户没有选择任何RadioButton,可以在这里进行提示
Toast.makeText(context, "请选择一个选项", Toast.LENGTH_SHORT).show();
} else {
// 根据用户选择的RadioButton进行下一步处理
// ...
}
}
});
// 添加取消按钮
builder.setNegativeButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// 处理用户点击取消按钮的事件
// ...
}
});
// 创建AlertDialog对象并显示
AlertDialog dialog = builder.create();
dialog.show();
```
需要在`R.layout.dialog_layout`布局文件中添加两个TextView和四个RadioButton,布局如下:
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="问题1" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="请选择一个选项:" />
<RadioButton
android:id="@+id/radioButton1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项1" />
<RadioButton
android:id="@+id/radioButton2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项2" />
<RadioButton
android:id="@+id/radioButton3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项3" />
<RadioButton
android:id="@+id/radioButton4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项4" />
</LinearLayout>
```
其中,`android:text`属性可以根据需要进行修改。
阅读全文
相关推荐
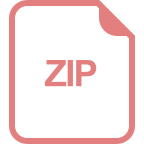








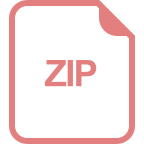
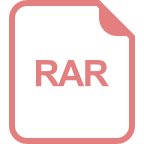
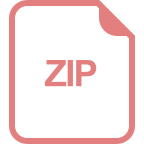
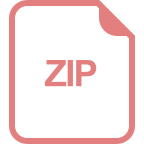



