java 将 list 转为 telegram bot excel 发送
时间: 2024-04-05 17:30:59 浏览: 318
要将 Java List 对象转换为 Excel 文件,并将其发送到 Telegram Bot,你可以使用 Apache POI 库来创建 Excel 文件,使用 Telegram Bot API 发送文件。
以下是一个示例代码,它将 Java List 对象转换为 Excel 文件,并将其发送到 Telegram Bot:
```java
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.telegram.telegrambots.meta.api.methods.send.SendDocument;
import org.telegram.telegrambots.meta.api.objects.InputFile;
import org.telegram.telegrambots.meta.bots.AbsSender;
import org.telegram.telegrambots.meta.exceptions.TelegramApiException;
public class ListToExcelSender {
public void send(List<Object[]> data, String chatId, AbsSender bot) throws IOException, TelegramApiException {
// Create the Excel file
XSSFWorkbook workbook = new XSSFWorkbook();
org.apache.poi.ss.usermodel.Sheet sheet = workbook.createSheet("Sheet1");
int rowNum = 0;
for (Object[] rowData : data) {
Row row = sheet.createRow(rowNum++);
int colNum = 0;
for (Object field : rowData) {
Cell cell = row.createCell(colNum++);
if (field instanceof String) {
cell.setCellValue((String) field);
} else if (field instanceof Integer) {
cell.setCellValue((Integer) field);
} else if (field instanceof Double) {
cell.setCellValue((Double) field);
} else if (field instanceof Boolean) {
cell.setCellValue((Boolean) field);
}
}
}
// Write the Excel file to a byte array
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
workbook.write(outputStream);
// Create a SendDocument request with the Excel file
SendDocument request = new SendDocument(chatId, new InputFile(outputStream.toByteArray(), "data.xlsx"));
// Send the Excel file to the Telegram Bot
bot.execute(request);
}
}
```
在上面的代码中,我们使用 `XSSFWorkbook` 类来创建一个新的 Excel 工作簿,然后创建一个名称为 "Sheet1" 的工作表。接着,我们遍历 Java List 对象中的数据,将它们添加到 Excel 表格中。最后,我们将 Excel 文件写入 ByteArrayOutputStream 中,并使用 Telegram Bot API 发送文件。
你可以使用以下代码将 Java List 对象转换为 Excel 文件,并将其发送到 Telegram Bot:
```java
List<Object[]> data = ... // 获取你要转换的数据
String chatId = "123456789"; // 指定要发送到的 Telegram 聊天 ID
AbsSender bot = ... // 创建你的 Telegram Bot 实例
ListToExcelSender sender = new ListToExcelSender();
sender.send(data, chatId, bot);
```
注意,在上面的代码中,我们假设你已经创建了一个 Telegram Bot 实例,并将其传递给 `sender.send()` 方法中。如果你还没有创建 Telegram Bot,请先参考 Telegram Bot API 的文档。
阅读全文
相关推荐
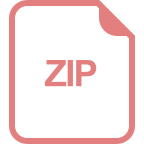
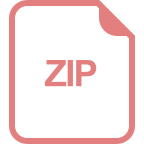
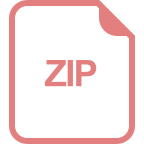
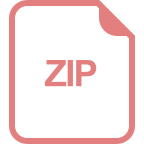
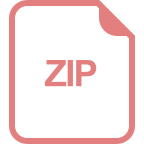



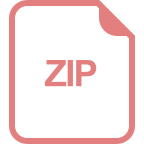
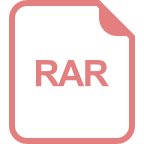
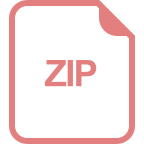
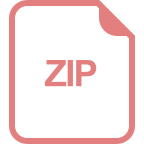
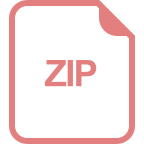
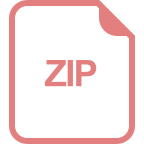
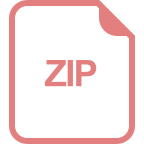
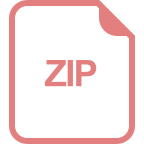
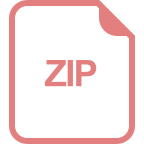