深度强化学习代码gpt
时间: 2023-09-21 19:02:53 浏览: 75
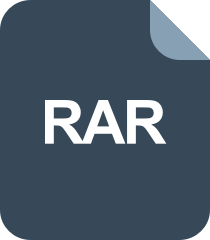
深度学习代码
以下是使用PyTorch实现GPT-2模型的示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class GPT2(nn.Module):
def __init__(self, vocab_size, n_embd, n_layer, n_head, n_positions):
super(GPT2, self).__init__()
self.n_embd = n_embd
self.n_layer = n_layer
self.n_head = n_head
self.n_positions = n_positions
self.vocab_size = vocab_size
self.wte = nn.Embedding(vocab_size, n_embd)
self.wpe = nn.Embedding(n_positions, n_embd)
self.drop = nn.Dropout(0.1)
self.h = nn.ModuleList([Block(n_embd, n_head, n_positions) for _ in range(n_layer)])
self.ln_f = nn.LayerNorm(n_embd)
def forward(self, x, positions):
h = self.wte(x)
position_embed = self.wpe(positions)
h = h + position_embed
h = self.drop(h)
for block in self.h:
h = block(h)
h = self.ln_f(h)
logits = torch.matmul(h, self.wte.weight.t())
return logits
class Block(nn.Module):
def __init__(self, n_embd, n_head, n_positions):
super(Block, self).__init__()
self.ln1 = nn.LayerNorm(n_embd)
self.attn = Attention(n_embd, n_head, n_positions)
self.ln2 = nn.LayerNorm(n_embd)
self.mlp = MLP(n_embd)
def forward(self, x):
h = self.ln1(x)
h = self.attn(h)
x = x + h
h = self.ln2(x)
h = self.mlp(h)
x = x + h
return x
class Attention(nn.Module):
def __init__(self, n_embd, n_head, n_positions):
super(Attention, self).__init__()
self.c_attn = nn.Conv1d(n_embd, n_embd * 3, 1, bias=False)
self.c_proj = nn.Conv1d(n_embd, n_embd, 1, bias=False)
self.n_head = n_head
self.split_size = n_embd
self.scale = 1 / (n_embd // n_head) ** 0.5
self.n_positions = n_positions
def forward(self, x):
query, key, value = self.c_attn(x).chunk(3, dim=1)
query = self.split_heads(query)
key = self.split_heads(key)
value = self.split_heads(value)
a = torch.matmul(query, key.transpose(-1, -2)) * self.scale
a = F.softmax(a, dim=-1)
a = self.dropout(a)
o = torch.matmul(a, value)
o = self.merge_heads(o)
o = self.c_proj(o)
o = self.dropout(o)
x = x + o
return x
def split_heads(self, x):
batch_size, length, hidden_size = x.size()
x = x.view(batch_size, length, self.n_head, hidden_size // self.n_head)
x = x.transpose(1, 2)
return x
def merge_heads(self, x):
batch_size, _, length, hidden_size = x.size()
x = x.transpose(1, 2).contiguous()
x = x.view(batch_size, length, hidden_size * self.n_head)
return x
def dropout(self, x):
return F.dropout(x, p=0.1, training=self.training)
class MLP(nn.Module):
def __init__(self, n_embd):
super(MLP, self).__init__()
self.c_fc = nn.Conv1d(n_embd, n_embd * 4, 1)
self.c_proj = nn.Conv1d(n_embd * 4, n_embd, 1)
self.act = F.gelu
def forward(self, x):
h = self.act(self.c_fc(x))
h = self.c_proj(h)
return h
```
需要注意的是,以上代码是GPT-2模型的简化版本,实际应用中可能需要进行一些修改和优化。此外,使用深度强化学习训练GPT-2模型需要大量数据和计算资源,需要有充足的准备。
阅读全文
相关推荐
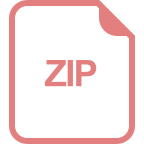
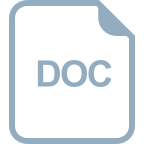
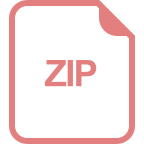
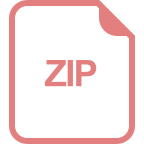
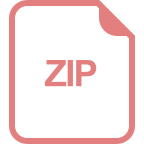
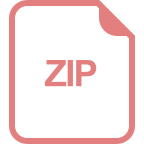
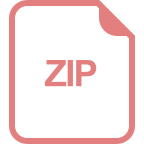
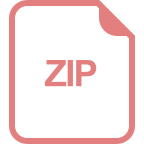
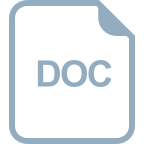
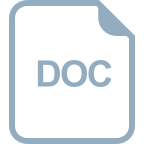
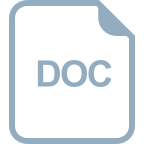
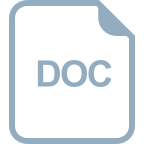
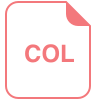
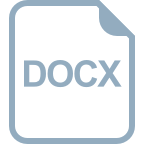