C语言写一个程序,完成文本文件的拷贝,即将当前目录下已经有的file1.txt拷贝一份为file2.txt文件(file2.txt拷贝时不存在)。
时间: 2024-02-16 18:59:55 浏览: 51
好的,您可以使用以下 C 代码来完成此任务:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *fp1, *fp2;
char ch;
// 定义文件名
char file1[] = "file1.txt";
char file2[] = "file2.txt";
// 判断 file2 是否存在,如果存在则退出程序
if ((fp2 = fopen(file2, "r")) != NULL) {
printf("%s already exists!\n", file2);
exit(1);
}
// 打开 file1 和 file2 文件,读取 file1 的内容并写入 file2
if ((fp1 = fopen(file1, "r")) == NULL) {
printf("Cannot open file %s!\n", file1);
exit(1);
}
if ((fp2 = fopen(file2, "w")) == NULL) {
printf("Cannot create file %s!\n", file2);
exit(1);
}
while ((ch = fgetc(fp1)) != EOF) {
fputc(ch, fp2);
}
// 关闭文件指针,拷贝完成
fclose(fp1);
fclose(fp2);
printf("%s has been copied to %s!\n", file1, file2);
return 0;
}
```
这段代码首先使用 `fopen()` 函数判断 `file2` 是否存在,如果存在则直接退出程序并提示信息;如果不存在,则打开 `file1` 和 `file2` 文件,读取 `file1` 的内容并写入到 `file2` 中,最后输出拷贝完成的提示信息。注意在写入文件时要使用 `fputc()` 函数逐个字符写入。最后需要使用 `fclose()` 函数关闭文件指针。
阅读全文
相关推荐
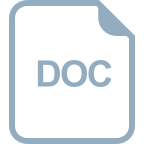
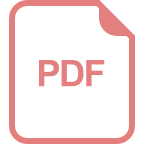
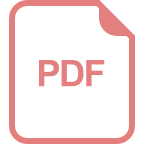















