设计一个复数Complex类,包括实部和虚部,能够实现+(成员函数重载),++(成员函数重载,包括前缀和后缀方式),能够实现转换为整数操作(整数值等于实部的值),并重载输出运算符。并给出测试程序。
时间: 2024-09-13 12:15:12 浏览: 38
设计一个名为`Complex`的类,用于表示复数,可以按照以下步骤实现:
```cpp
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
// 构造函数
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
// + 运算符重载(加法)
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 自增运算符重载(前缀和后缀)
Complex& operator++() { // 前缀++
real++;
imag++;
return *this;
}
Complex operator++(int) { // 后缀++
Complex temp(*this);
++*this;
return temp;
}
// 转换为整数(只取实部)
operator int() const {
return static_cast<int>(real);
}
// 输出运算符重载
friend std::ostream& operator<<(std::ostream& os, const Complex& c) {
os << c.real << " + " << c.imag << "i";
return os;
}
// 测试程序
void test() {
Complex a(1.5, 2.0);
Complex b(3.0, -4.5);
Complex sum = a + b;
std::cout << "Sum: " << sum << std::endl;
Complex increment_a = a;
for (int i = 0; i < 5; ++increment_a) {
std::cout << "Incremented a: " << increment_a << std::endl;
}
std::cout << "a as an integer: " << a << std::endl;
}
};
```
在上述代码中,我们定义了一个`Complex`类,并实现了加法、自增运算符、转换为整数以及输出运算符的重载。`test()`函数作为示例展示了如何创建复数对象并进行一些基本操作。
阅读全文
相关推荐
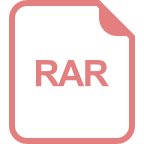
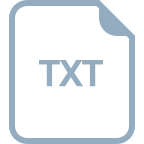
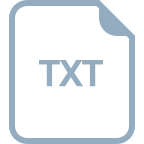



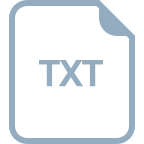
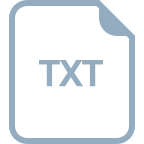
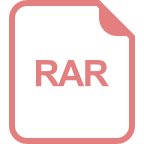
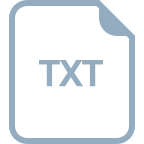
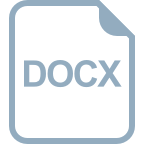
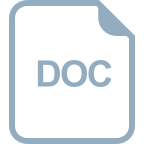
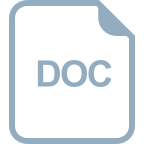
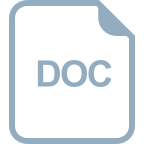
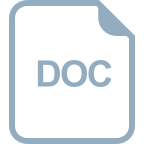
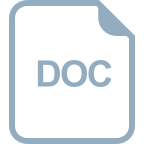
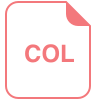
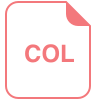