点击表格内的编辑 弹出模态框 模态框里有下拉选个人和企业 选择个人模态框表格展示个人内容 选择企业 模态框表格展示企业内容 选中一条数据 点击保存 将编辑的数据改为选中的这条 用React类组件加Antd实现
时间: 2024-06-08 13:11:57 浏览: 134
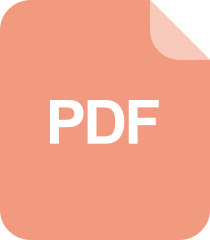
vue+element-ui实现表格编辑的三种实现方式
首先,我们需要在代码中引入Antd组件库以及相关的样式文件:
```javascript
import React, { Component } from 'react';
import { Modal, Table, Select, Button } from 'antd';
import 'antd/dist/antd.css';
```
然后,我们创建一个类组件,并在构造函数中初始化state:
```javascript
class EditTableModal extends Component {
constructor(props) {
super(props);
this.state = {
visible: false,
data: [],
selectedRowKeys: [],
selectedRows: [],
type: '',
};
}
// ...
}
```
接下来,我们需要实现打开和关闭模态框的方法:
```javascript
showModal = () => {
this.setState({
visible: true,
});
};
handleCancel = () => {
this.setState({
visible: false,
});
};
```
在模态框内部,我们需要渲染一个下拉框和一个表格。当用户选择下拉框中的选项时,表格会动态显示对应的内容。
```javascript
render() {
const { visible, data, selectedRowKeys, type } = this.state;
const columns = [
{ title: '姓名', dataIndex: 'name', key: 'name' },
{ title: '年龄', dataIndex: 'age', key: 'age' },
{ title: '地址', dataIndex: 'address', key: 'address' },
];
return (
<div>
<Button onClick={this.showModal}>编辑</Button>
<Modal title="编辑" visible={visible} onCancel={this.handleCancel} footer={null}>
<Select defaultValue="请选择" style={{ width: 120 }} onChange={this.handleTypeChange}>
<Option value="person">个人</Option>
<Option value="company">企业</Option>
</Select>
{type === 'person' && (
<Table
columns={columns}
dataSource={data}
rowSelection={{
selectedRowKeys,
onChange: this.handleSelectChange,
}}
/>
)}
{type === 'company' && (
<Table
columns={columns}
dataSource={data}
rowSelection={{
selectedRowKeys,
onChange: this.handleSelectChange,
}}
/>
)}
</Modal>
</div>
);
}
```
当用户选择下拉框中的选项时,我们需要更新state中的type字段,并根据type字段动态获取表格数据。
```javascript
handleTypeChange = value => {
let data = [];
if (value === 'person') {
data = [
{ key: 1, name: '张三', age: 18, address: '北京市海淀区' },
{ key: 2, name: '李四', age: 20, address: '上海市浦东新区' },
{ key: 3, name: '王五', age: 22, address: '广州市天河区' },
];
} else if (value === 'company') {
data = [
{ key: 1, name: '阿里巴巴', age: 20, address: '杭州市西湖区' },
{ key: 2, name: '腾讯', age: 22, address: '深圳市南山区' },
{ key: 3, name: '百度', age: 18, address: '北京市海淀区' },
];
}
this.setState({
data,
type: value,
selectedRowKeys: [],
selectedRows: [],
});
};
```
当用户选择表格中的数据时,我们需要更新state中的selectedRowKeys和selectedRows字段。
```javascript
handleSelectChange = (selectedRowKeys, selectedRows) => {
this.setState({ selectedRowKeys, selectedRows });
};
```
最后,用户点击保存按钮时,我们需要将编辑的数据改为选中的数据,并关闭模态框。
```javascript
handleSave = () => {
const { selectedRows } = this.state;
// 将编辑的数据改为选中的数据
console.log(selectedRows);
// 关闭模态框
this.handleCancel();
};
```
完整的代码如下:
```javascript
import React, { Component } from 'react';
import { Modal, Table, Select, Button } from 'antd';
import 'antd/dist/antd.css';
const { Option } = Select;
class EditTableModal extends Component {
constructor(props) {
super(props);
this.state = {
visible: false,
data: [],
selectedRowKeys: [],
selectedRows: [],
type: '',
};
}
showModal = () => {
this.setState({
visible: true,
});
};
handleCancel = () => {
this.setState({
visible: false,
});
};
handleTypeChange = value => {
let data = [];
if (value === 'person') {
data = [
{ key: 1, name: '张三', age: 18, address: '北京市海淀区' },
{ key: 2, name: '李四', age: 20, address: '上海市浦东新区' },
{ key: 3, name: '王五', age: 22, address: '广州市天河区' },
];
} else if (value === 'company') {
data = [
{ key: 1, name: '阿里巴巴', age: 20, address: '杭州市西湖区' },
{ key: 2, name: '腾讯', age: 22, address: '深圳市南山区' },
{ key: 3, name: '百度', age: 18, address: '北京市海淀区' },
];
}
this.setState({
data,
type: value,
selectedRowKeys: [],
selectedRows: [],
});
};
handleSelectChange = (selectedRowKeys, selectedRows) => {
this.setState({ selectedRowKeys, selectedRows });
};
handleSave = () => {
const { selectedRows } = this.state;
// 将编辑的数据改为选中的数据
console.log(selectedRows);
// 关闭模态框
this.handleCancel();
};
render() {
const { visible, data, selectedRowKeys, type } = this.state;
const columns = [
{ title: '姓名', dataIndex: 'name', key: 'name' },
{ title: '年龄', dataIndex: 'age', key: 'age' },
{ title: '地址', dataIndex: 'address', key: 'address' },
];
return (
<div>
<Button onClick={this.showModal}>编辑</Button>
<Modal title="编辑" visible={visible} onCancel={this.handleCancel} footer={null}>
<Select defaultValue="请选择" style={{ width: 120 }} onChange={this.handleTypeChange}>
<Option value="person">个人</Option>
<Option value="company">企业</Option>
</Select>
{type === 'person' && (
<Table
columns={columns}
dataSource={data}
rowSelection={{
selectedRowKeys,
onChange: this.handleSelectChange,
}}
/>
)}
{type === 'company' && (
<Table
columns={columns}
dataSource={data}
rowSelection={{
selectedRowKeys,
onChange: this.handleSelectChange,
}}
/>
)}
<Button onClick={this.handleSave}>保存</Button>
</Modal>
</div>
);
}
}
export default EditTableModal;
```
阅读全文
相关推荐
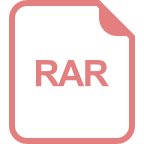
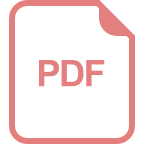
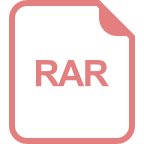
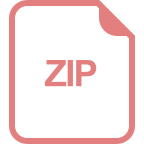
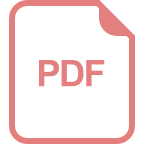
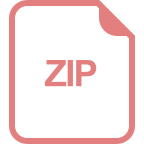
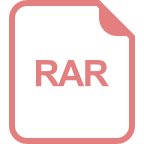
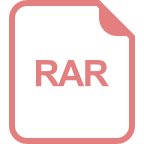
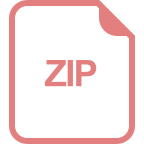
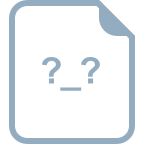
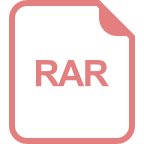
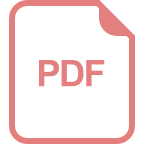
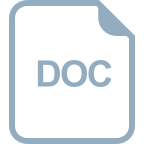
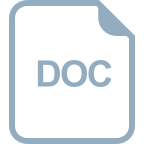
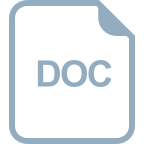
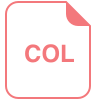
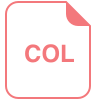
